React Crash Course for Beginners - Learn ReactJS from Scratch in this 100% Free Tutorial!
Academind・2 minutes read
Maximilian Schwartzmuller's React course covers building user interfaces, emphasizing the benefits of React's component-based approach for efficient web development. React simplifies UI construction with manageable components and a declarative nature, enhancing user experience by making web apps more dynamic and responsive.
Insights
- React is a JavaScript library designed for building user interfaces in web browsers, allowing for dynamic page manipulation without reloading, enhancing user experience compared to traditional web apps.
- React simplifies web development by breaking applications into manageable components, promoting code maintainability and a declarative approach that focuses on end results rather than low-level details.
- React enables the creation of custom HTML elements, preferred for single-page applications to provide a smooth user experience controlled by JavaScript.
- Setting up a React project involves tools like Create React App, requiring Node.js installation, to automate configurations and provide a development server for browser compatibility.
- React components, acting as custom HTML elements, can be composed, styled, and conditionally rendered using props, state management, and hooks like useState for dynamic interfaces.
Get key ideas from YouTube videos. It’s free
Recent questions
What is React?
A JavaScript library for building user interfaces.
How can I set up a React project?
Use Create React App for automated configurations.
What are React components?
Building blocks for user interfaces.
How can I handle state in React components?
Use the useState hook for dynamic changes.
What is the purpose of React Context?
Manage application-wide state conveniently.
Related videos
Programming with Mosh
React Tutorial for Beginners
Academind
Get started with React.js & React Router 6+
Traversy Media
React Crash Course 2024
Honeypot
How A Small Team of Developers Created React at Facebook | React.js: The Documentary
JavaScript Mastery
React JS Full Course 2023 | Build an App and Master React in 1 Hour
Summary
00:00
"React Course: Simplifying Web Development with Components"
- Maximilian Schwartzmuller is the instructor of a course on React, a popular JavaScript library for building user interfaces.
- The course includes building a complete example project to learn key features of React step by step.
- React is a client-side JavaScript library specifically for building user interfaces in web browsers.
- JavaScript in the browser allows for dynamic manipulation of web pages without requesting new HTML pages, resulting in a smoother user experience.
- Traditional web apps required loading new HTML pages, causing delays, unlike the reactive experience of mobile apps.
- Using just JavaScript for web development can become complex and repetitive, especially when handling multiple elements or dynamic content.
- React simplifies building user interfaces by breaking down applications into manageable components, making code more maintainable.
- React's declarative approach allows developers to focus on defining the desired end result rather than low-level implementation details.
- React enables the creation of custom HTML elements, making it easier to build modern, rich user interfaces.
- React is preferred for single-page applications, where JavaScript controls all aspects of the user interface, providing a smoother user experience.
19:07
Setting up React project with Create React App
- To set up a React project, the popular tool Create React App is recommended, which automates the necessary configurations.
- Node.js must be installed before using Create React App, as it is required for running JavaScript outside the browser.
- Visit nodejs.org to install the latest version of Node.js for your operating system, following the installation process.
- To create a React project, open the terminal, navigate to the desired folder, and run the command "npx create-react-app [project-name]".
- The project setup includes an automatically reloading development server and code transformations for browser compatibility.
- After running the command, a new folder with the project name will be created, containing a basic React project setup.
- Open the project folder in a code editor like Visual Studio Code, ensuring to install the Prettier extension for code formatting.
- Remove unnecessary starting files like logo, tests, and CSS files from the project to start fresh with your own code.
- Install third-party dependencies by running "npm install" in the terminal to download and store the required packages.
- Start the development server with "npm start" to view the React project in the browser, ensuring to keep the server running for code changes to reflect instantly.
37:41
"Building React Components for Dynamic Web"
- React applications are typically built with a single HTML file fetched from a server, with React controlling the DOM.
- In the index.html file, a div with the id "root" is present, where the app HTML element from app.js is rendered.
- The app element in app.js is a function that returns JSX code, making it a React component.
- React components are functions returning JSX, acting as custom HTML elements defined by developers.
- Custom elements created in React are not recognized by the browser; instead, React renders the content returned by these elements.
- To enhance the appearance of a component, styling can be added through CSS classes in the index.css file.
- Components in React are building blocks that can be composed together for flexibility and reusability.
- Components in React must start with a capital character when used as custom HTML elements.
- Data can be passed into components using props, allowing for configurability and dynamic content.
- By utilizing props, different data can be passed into components, enabling the display of varied content based on the provided values.
56:40
"React components manage data, events, states"
- React uses the concept of props, which are key-value pairs stored in a JavaScript object, to pass data to components.
- In JSX code, dynamic expressions can be included using curly braces, allowing for JavaScript evaluation within the code.
- Components in React can listen to events like clicks by adding attributes like `onClick` to elements, triggering functions when the event occurs.
- To handle events in React, functions can be defined within components and referenced in event attributes like `onClick`.
- React components can be split into separate files for better organization, with each component handling specific functionalities.
- Components like modals and backdrops can be created in separate files and imported into the main app file for use.
- React components can manage states using the `useState` hook, allowing for dynamic changes in the interface based on state changes.
- The `useState` hook in React returns an array with two elements, enabling the storage of the current state and a function to update it.
- By utilizing the `useState` hook, React components can control the visibility of elements like modals based on state changes.
- React components can dynamically render different outputs based on the current state, enabling interactive and responsive user interfaces.
01:16:17
Understanding React State and Rendering Components
- React state consists of two elements: a state snapshot and a function to change the state.
- The first element stores the current state value, acting like a variable managed by React.
- The second element is a function used to update the state value.
- State changes trigger a re-execution of the component function, updating the rendered content.
- To conditionally render components, use state values like modal is open.
- Components can be conditionally rendered using dynamic expressions in JSX.
- Use the logical AND operator (&&) for conditional rendering in a shorter form.
- Custom components require explicit configuration for event listeners like onClick.
- Functions can be passed as props to components for execution.
- Routing in React involves adding a router tool to handle URL changes and component loading.
01:35:33
"React Router: Components, Navigation, CSS Modules"
- Components in React need to be exported and return JSX code.
- The router package is used to define which page should be loaded based on URLs.
- The BrowserRouter component is used to wrap the app component in index.js.
- The Route component from react-router-dom is used to define paths and components to load.
- Different page components are imported and used within the Route component.
- The Switch component is used to ensure only one route is active at a time.
- The exact prop is used to match the full path instead of just the beginning.
- A main navigation component is created to add navigation links.
- Links are created using the Link component from react-router-dom to prevent unnecessary server requests.
- CSS modules are used to scope styles to specific components by importing classes from CSS files.
01:56:03
Building React Meetup Page with Navigation Bar
- Added a navigation bar with component-specific styles in React.
- Started working on the "all meetups" page by creating dummy meetup data.
- Created an array of dummy meetups with details like image, title, description, and address.
- Demonstrated how to render a list of JSX elements dynamically using the map method in React.
- Emphasized the importance of adding a unique key prop to list items in React to avoid warnings.
- Introduced the concept of creating reusable components for meetups, including a meetup item and meetup list.
- Showed how to pass dynamic data to the meetup item component using props in React.
- Implemented styling for the meetup item component using CSS modules.
- Created a separate UI component, a card, to provide a consistent card-like design for components.
- Illustrated how to use the card component to wrap JSX content for a card-like appearance in React components.
02:15:54
"React Components, Layouts, and Meetups Data"
- JSX content in React components is stored in the children prop, which holds the content between component tags.
- Using the card component in a component allows for displaying the content stored in children prop.
- Wrapping components with a div with a card class is a technique for composing user interfaces in React.
- Creating a layout component involves adding a layout module CSS file and using props.children to render content.
- Utilizing a layout component helps in structuring the application layout and removing extra code from the app component.
- The goal is to have meetups data stored on a server to be shared globally and not lost on page reload.
- Adding a form to the add new meetup page is the first step towards gathering and sending meetup data to a server.
- The new meetup form component is created in a separate file within the meetups folder.
- The form includes inputs for meetup title, image URL, address, and description, styled using CSS classes.
- Handling form submission involves preventing the browser default behavior and using refs to read entered values for each input.
02:37:10
Sending Meetup Data to Firebase Database
- The address and description are obtained using ref.current.value for the address input and description input, respectively.
- A new object, meetup data, is created to store the entered title, image, address, and description.
- The meetup data is logged with console.log for testing purposes before being sent to a server.
- The goal is to send the meetup data to a server, but no server-side code is written in this front-end focused course.
- Firebase is used as a dummy backend to store the data in a database, as connecting a front-end application directly to a database is insecure.
- Firebase offers a real-time database service with an API to send requests and store data.
- The URL for sending requests to Firebase includes adding ".json" at the end and using a post request to store data.
- The use of the fetch function in JavaScript allows sending HTTP requests, with JSON data typically being sent in the body.
- The use of the useHistory hook from React Router allows for programmatically navigating away from a page after a successful request.
- To load the stored meetups from Firebase into the application, a HTTP request is sent when the all meetups page component is rendered.
02:56:49
Handling Data and State in React Components
- When using fetch to get data, the response is handled using the then method, passing an anonymous function to access the response object automatically.
- To read the body of the response data and convert it to a JavaScript object, the json method is used, which returns a promise that needs to be resolved.
- Error handling can be added in a separate then block to work on the data received from the response.
- To avoid returning a promise in a React component function, temporary JSX code like a loading spinner can be returned until the response data is received.
- State can be used to update what is displayed on the screen based on certain conditions, such as showing loading content until data is fetched.
- To prevent an infinite loop caused by state updates triggering component re-renders, the useEffect hook can be used to control when certain code, like fetching data, should run.
- The useEffect hook takes an array of dependencies to specify when the code inside it should run, ensuring it doesn't execute unnecessarily.
- When fetching data from an API like Firebase, where the response is an object with nested data, the data needs to be transformed into an array before setting the state.
- By transforming the data fetched from Firebase into an array of meetups, the data can be properly displayed in the component without causing issues.
- To manage state that affects multiple components, a mechanism for global state management, like lifting the state up to a higher-level component, may be necessary to ensure consistent data handling across the application.
03:16:54
Efficient State Management with React Context
- Passing the number of favorites to the app component and the array of favorite meetups to the favorites page component is a common practice.
- Managing state in the app component and distributing it through props can lead to downsides like increasing complexity and longer prop chains.
- State management solutions like Redux or React's built-in Context feature can help manage application-wide state more conveniently.
- Setting up the Context feature involves creating a new folder named "store" and adding a "favoritesContext.js" file.
- Using the "createContext" function from React helps create a context object, which contains a React component.
- The "favoritesContext" object includes an initial value for the context, such as an object with keys like "favorites" and "totalFavorites."
- The "favoritesContextProvider" component is responsible for providing the context to interested components and updating context values.
- Managing state in the "favoritesContextProvider" involves functions like "addFavoriteHandler" and "removeFavoriteHandler" to add or remove favorites.
- The use of the "useState" hook helps manage state dynamically and ensures the latest state snapshot is used for updates.
- Exposing functions like "addFavorite" and "removeFavorite" from the context allows other components to interact with and update the context values.
03:37:48
Managing Meetup Favorites with React Context
- To determine if a meetup item is part of the context, use the useContext hook provided by React, passing the context object as an argument.
- The favorites context object, created with createContext, is accessed by importing the favorites context in the MeetupItem component.
- To check if a meetup item is a favorite, use the itemIsFavorite method defined in the context, passing the meetup id obtained from props.
- In the toggleFavoriteStatusHandler, if the item is a favorite, remove it from the context using the removeFavorite method with the meetup id.
- If the item is not a favorite, add it to the context using the addFavorite method, passing a new meetup object constructed with props data.
- On the My Favorites page, import useContext and the favorites context object to display a list of favorite meetups from the context.
- To handle cases where there are no favorites, create a content variable to display a message if total favorites are zero, else display the meetup list.

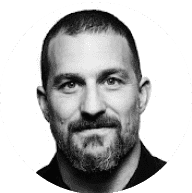
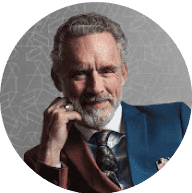
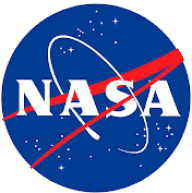
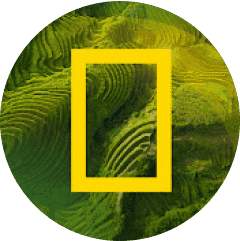