Get started with React.js & React Router 6+
Academind・170 minutes read
React.js is a popular library for building reactive user interfaces that simplifies code and enhances user experience through blending HTML and JavaScript. The video covers key concepts, including project setup, component creation, styling, managing states, form handling, and routing, necessary for React developers.
Insights
- React.js simplifies coding for interactive websites, improving user experience by blending HTML and JavaScript code for readability.
- React.js enables declarative code writing, streamlining UI development through features like JSX and reusable components.
- React's useState hook is pivotal for managing state changes and triggering UI updates, ensuring components reflect the latest data accurately.
- React Router is crucial for implementing different paths and loading components based on those paths, enhancing navigation in React applications.
- Leveraging React Router's advanced features like data loading and submission simplifies code complexity and boosts efficiency in React projects.
- Implementing dynamic routes in React Router allows for flexible navigation and rendering components based on dynamic path parameters, enhancing the user experience.
Get key ideas from YouTube videos. It’s free
Recent questions
What is React.js?
React.js is a JavaScript library for building reactive user interfaces. It simplifies code for interactive websites, enhances user experience, and allows blending HTML and JavaScript code for readability and manageability.
How is a React project set up?
Creating a React project requires tools like Create React App or Vite, necessitating Node.js installation. The project setup transforms code for browser compatibility and provides a live preview for development. JSX files contain HTML code blended with JavaScript, with the main .jsx file serving as the entry point.
What are components in React?
Components in React are functions that return JSX code, serving as building blocks for composing complex user interfaces. Custom components, like Post, are created in separate files and imported for use in JSX code. Components must start with an uppercase character and can be reused multiple times.
How does React handle state?
React uses the useState hook to manage state changes and trigger UI updates. By calling the state updating function, React re-renders the component to reflect the new state. State can be lifted up from one component to another for shared access, ensuring proper data display and manipulation.
What is React Router?
React Router is essential for supporting different paths and loading various components based on those paths in React applications. Route configurations are set up to define paths and components to render, enabling routing for different pages. Layout routes can be created to share layout elements in complex apps, enhancing navigation and user experience.
Related videos
JavaScript Mastery
React JS Full Course 2023 | Build an App and Master React in 1 Hour
Programming with Mosh
React Tutorial for Beginners
Web Dev Simplified
Learn React Router v6 In 45 Minutes
IDStack
Satu Jam Jago React.js Hook Basic - Tutorial React.js Indonesia
Web Dev Simplified
Learn useReducer In 20 Minutes
Summary
00:00
"Master React.js for Reactive User Interfaces"
- React.js is a popular JavaScript library for building highly reactive user interfaces.
- The video provides a foundational understanding of React.js, essential for React developers.
- Key concepts are explored through building a demo application in the video.
- React.js simplifies code for interactive websites, enhancing user experience.
- React.js allows blending HTML and JavaScript code, making code more readable and manageable.
- React.js enables declarative code writing, simplifying UI development.
- Creating a React project requires tools like Create React App or Vite, necessitating Node.js installation.
- The project setup transforms code for browser compatibility and provides a live preview for development.
- JSX files in the project contain HTML code blended with JavaScript, transformed for browser execution.
- The main .jsx file serves as the entry point, importing features from React and ReactDOM libraries for website functionality.
17:17
"React Library and ReactDOM in Action"
- React is formed by two separate libraries, React Library and ReactDOM.
- In main JSX, ReactDOM's createRoot method is called to render React code.
- The createRoot method points to an element in the HTML code using Vanilla JavaScript.
- The StrictMode feature in React provides extra checks for code quality.
- The App component is imported and rendered in main JSX, displaying JSX code.
- Components in React are functions that return JSX code.
- Components are building blocks used to compose complex user interfaces.
- A custom component, Post, is created in a separate file, Post.jsx.
- The Post component is imported and used in the App component's JSX code.
- Custom components in JSX code must start with an uppercase character.
35:39
"Reusable React Components with Props and Styling"
- The built-in random method in JavaScript can be used to select between two names based on a condition.
- React treats JavaScript code as components, which are essentially functions executed by React.
- JSX syntax in React allows for dynamic values to be output by executing JavaScript expressions within curly braces.
- Components can be reused multiple times in JSX code, with each instance being executed separately by React.
- JSX code must have a single root element, with multiple sibling elements wrapped within it.
- Components with no content between opening and closing tags can be written as self-closing tags in JSX.
- Props in React allow for custom attributes to be passed to components, enabling dynamic content based on these attributes.
- Props are accessed within a component function as an object, with custom attributes set on the component being accessible through this object.
- Styling components in React can be done by adding the style attribute with a JavaScript object as a value, rather than inline styles as strings.
- Reusable components with props and styling enhance the flexibility and visual appeal of React applications.
53:10
Styling and Component Composition in React
- Inline styles can be set in JSX by passing an object to the style prop, with properties like color and text align.
- It's recommended to avoid inline styles and instead use CSS files for styling in React projects.
- JSX uses a JavaScript version of HTML, with some attribute names differing, like using className instead of class for CSS classes.
- To avoid naming clashes in larger projects, CSS styles can be scoped to components using CSS modules.
- CSS modules transform class names to unique ones, preventing clashes, and are activated by naming CSS files with .module.css extension.
- Unique class names from CSS modules are imported into components and applied using the class name prop.
- A PostsList component is created to render a list of posts using CSS modules for styling.
- The PostsList component is imported and used in the App component, showcasing component composition in React.
- The NewPost component is introduced for adding new posts, with a form for entering text and author name.
- NewPost component is imported and used in the App component, with adjustments made to ensure a single root element in JSX.
01:11:51
Managing Dynamic Website States with React.useState
- Websites have different states, especially dynamic ones where content changes after loading.
- React helps manage these states to update the user interface accordingly.
- To update text based on user input, two main steps are required: registering a state and setting up an event listener.
- In React, event listeners are added using special props like onKeyDown or onChange.
- Functions can be defined within component functions to handle events like text changes.
- React's useState hook is crucial for managing state changes and triggering UI updates.
- useState returns an array with the current state value and a function to update it.
- By calling the state updating function, React re-renders the component to reflect the new state.
- Using array destructuring, the current state value and updating function can be easily accessed.
- Placing state in the appropriate component, like PostsList, ensures the entered text is displayed correctly in the UI.
01:30:21
Managing State and Props in React Components
- To move state from NewPost to PostsList, import useState in PostsList and register the state there, using object destructuring to access enteredBody and setEnteredBody.
- Lifting state up involves moving state manipulated in one component to another component that needs it, allowing access to both components requiring the state.
- To implement lifting state up, remove state and event handling from NewPost, and use props to pass functions like onBodyChange to handle state manipulation.
- Functions can be passed as values to props, like bodyChangeHandler, to handle events and update state in the component where needed.
- Multiple state values can be managed in a component by calling useState multiple times, allowing for separate state slices like enteredAuthor.
- Updating state values triggers re-execution of the component function, reflecting updated values in the UI by passing them as props to nested components.
- To create a modal overlay, a new Modal component is added, wrapping content like NewPost to achieve the overlay look.
- The children prop is used to pass content between opening and closing tags of a custom component, allowing React to render the wrapped content inside the component.
- Adding an open prop to the dialogue element ensures visibility of the modal, while styling adjustments help achieve the desired overlay effect.
- To close the modal by clicking on the backdrop, manage a state slice like modalIsVisible in PostsList, updating it based on events like clicking on the backdrop in the Modal component.
01:48:45
Managing Modal Visibility in React Components
- To hide the modal when the backdrop is clicked, a new function called hideModalHandler is added to setModalIsVisible to false as a new state value.
- The hideModalHandler function is passed as a value to a prop called onClose in the modal component without parentheses to ensure the function itself is passed.
- The onClose prop in the modal component is destructured and set as a value to onClick to link the click listener to the hideModalHandler function.
- Conditional rendering is implemented to show the modal content only if modalIsVisible is true, using a ternary expression.
- An alternative approach involves storing the modal content in a variable and rendering it based on the value of modalIsVisible.
- Another method uses the logical AND operator to render content based on the truthiness of modalIsVisible.
- To open the modal again, a MainHeader component with a button is added above the PostsList, requiring the installation of the React icons library.
- The state controlling the modal visibility is lifted up from the PostsList component to the App component using useState and functions like hideModalHandler and showModalHandler.
- The information about modal visibility is passed down to the PostsList component using props, with functions like onCreatePost and onStopPosting facilitating the opening and closing of the modal.
- Buttons for submitting and canceling in the NewPost component are added, with the cancel button set to type button to prevent form submission and linked to the hideModalHandler function through props.
02:06:07
"Enhancing Form Submission in React Components"
- To handle form submission, an event listener called onSubmit should be added to the NewPost component's form element to listen to the default submit event.
- The function executed when the submit event occurs should collect the entered values, which are now managed in the NewPost component.
- State slices should be brought back to the NewPost component by importing useState from React and removing them from the parent component, PostsList.
- The onBodyChange and onAuthorChange functions in the NewPost component should be removed, and the bodyChangeHandler should be called when the textarea changes.
- A submitHandler function should be added to be triggered when the form is submitted, preventing the default behavior of generating and sending an HTTP request.
- The entered body and author values should be grouped into a postData object, which can be added to a list of posts and rendered dynamically as a list of Post components.
- The addPostHandler function should be added to the PostsList component to update the list of posts when a new post is added.
- The addPostHandler function should be executed when the form is submitted, passing the postData to update the list of posts.
- The map method should be used on the posts array in the PostsList component to transform each post object into a Post JSX element, ensuring each list item has a unique key prop.
- Conditional rendering should be implemented in the PostsList component to display a message if there are no posts yet, showing a message to start adding posts.
02:23:58
"Essential React features for building interfaces"
- Fallback text disappears when a NewPost is added, revealing a list of posts.
- Key concepts for working with React are essential to know.
- Time to explore more advanced features in React.
- Understanding essential React features for building reactive user interfaces.
- Demo app only functions in the browser, data is lost upon reload.
- React is a front-end JavaScript library for building user interfaces.
- React is used to create single-page applications where JavaScript powers the interface.
- Need for a backend to store data from the React app.
- Backend API can be built with any language or framework.
- Link provided for a dummy backend project built with Node and Express.js.
02:40:51
"React useEffect and Router for Component Control"
- React's useEffect function controls when a function executes indirectly, ensuring it doesn't always run with the component function.
- The useEffect function prevents infinite loops by executing only when specific dependencies change, specified in an array passed to useEffect.
- An empty array as a dependency means the function executes once when the component first renders.
- The useEffect hook fetches data when a component is first rendered, providing fine-grain control over execution.
- To handle loading states while fetching data, a fetching state can be added using useState to show loading text or posts based on the fetching status.
- Error handling for responses can be added to display error messages if needed.
- React Router is essential for supporting different paths and loading various components based on those paths in React applications.
- React Router DOM package must be installed to enable routing in the front-end application.
- Route configurations are set up using createBrowserRouter to define paths and components to render for each path.
- Multiple routes can be added to support different pages like the starting page, new post page, and post detail page, each with its own path and component to render.
02:57:23
Creating Layout Routes in React Router
- React Router offers the feature of creating layout routes for shared layout elements in complex React apps.
- Layout routes are normal routes that nest other routes inside them to share layout elements like a main navigation bar.
- To create a layout route, a new route definition is added with a path of slash nothing to wrap all routes.
- A RootLayout JSX file is created in a routes folder to organize components, containing a MainHeader and other elements.
- The RootLayout route is imported into the main JSX file and nested route definitions are added to share the layout.
- The outlet component from React Router DOM is added to the RootLayout to render the content of nested routes.
- The app component is refactored to be a separate route in the routes folder, removing duplicate headers.
- The NewPost component is moved to the routes folder and loaded as a modal overlay using the modal component.
- The PostsList component is cleaned up to no longer handle adding NewPosts, with the NewPost component now responsible.
- The MainHeader component is updated to use the link component from React Router DOM to navigate without reloading the page.
03:13:14
Enhancing User Experience with React Router
- To ensure the cancel button closes the modal, navigate to the new post component where the cancel button is located.
- Import the link component from React Router DOM to replace the cancel button with a link for programmatic navigation.
- Remove the onClick event listener and onCancel prop as they are no longer needed.
- Specify the destination for the link to navigate to by adding the "to" prop with the desired route.
- Update the styling by replacing the content of the NewPost module CSS file with the provided updated CSS content.
- Implement routing to enable sharing URLs and linking different parts of the website, enhancing user experience.
- React Router version 6.4 or higher offers features beyond component routing, aiding in data fetching and submission.
- Utilize the loader property in route definitions to execute functions for data loading before rendering components.
- Use the useLoaderData hook in components to access data returned by the loader function assigned to the active route.
- Enhance user experience by leveraging React Router's features for data loading and submission, reducing code complexity and improving efficiency.
03:29:42
"React Router Form Data Submission Simplified"
- React Router will generate a request object with form data when a form is submitted.
- The method prop should be set to post in the Form component to align with HTTP semantics.
- The request object generated by React Router includes form data and a method that can be accessed in the action function.
- The formData method of the request object allows access to the data submitted in the form.
- Using object destructuring, the request object can be accessed in the action function.
- The formData object can be simplified using the Object class and the fromEntries method.
- The postData obtained from the form data can be used for sending requests.
- The redirect function from React Router DOM can be used to navigate to a different route after sending a request.
- Setting up a dynamic route with a placeholder for post details involves defining a route with a dynamic path parameter.
- The postDetails component can be rendered with loader data fetched using the loader function.

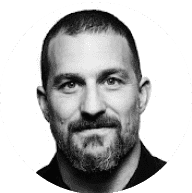
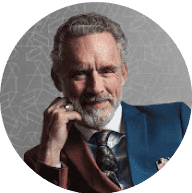
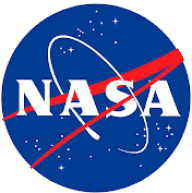
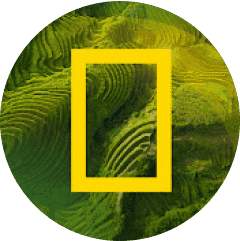