React Crash Course 2024
Traversy Media・2 minutes read
The React crash course covers building a job listing website using React 18 and 19, focusing on components, props, state, data fetching, routing, and tools like Vit and Json server. Key concepts include creating components, styling with Tailwind, managing state, data fetching with hooks, and deploying projects.
Insights
- React is a JavaScript library, not a framework, that allows for building dynamic user interfaces with components, maintained by Facebook and a community of developers.
- The React crash course covers fundamental concepts such as components, props, state, hooks, and data fetching, focusing on creating a front end for a job listing website using React Router and a mock REST API.
- Vit, a fast and efficient tool built on ES Build, is recommended for setting up React projects due to its speed, developer experience, and features like a development server with hot module replacement.
- Essential topics covered in the course include JSX syntax, dynamic rendering, component organization, data fetching, routing with React Router, and creating a full CRUD application with React, emphasizing efficient UI component organization and management.
Get key ideas from YouTube videos. It’s free
Recent questions
What is React?
React is a JavaScript library for building user interfaces, maintained by Facebook and a community of developers.
Related videos
Programming with Mosh
React Tutorial for Beginners
JavaScript Mastery
React JS Full Course 2023 | Build an App and Master React in 1 Hour
Academind
Get started with React.js & React Router 6+
Rocketseat
COMEÇANDO NO REACT.JS EM 2022
Academind
React Crash Course for Beginners - Learn ReactJS from Scratch in this 100% Free Tutorial!
Summary
00:00
2024 React Crash Course for Beginners
- The course is a 2024 React crash course, held every two to three years to stay updated on major frameworks.
- Geared towards beginners, the course aims to swiftly familiarize users with React, focusing on building a front end for a job listing website.
- Concepts covered include components, props, state, hooks, data fetching, and more.
- React Router is utilized to create a multi-page application.
- Json server library is employed to create a mock REST API for backend data.
- Upcoming React 19 introduces a compiler to enhance performance by converting React code into regular JavaScript.
- React 18 is used in the video, with core concepts remaining consistent between versions 18 and 19.
- React is a JavaScript library for building user interfaces, maintained by Facebook and a community of developers.
- React is a UI library, not a framework, allowing for the creation of dynamic user interfaces with components.
- React's virtual DOM and upcoming compiler enhance performance and ease of use, making it a popular choice for building various applications.
15:43
"Streamline React setup with Vit tool"
- Using a tool called Vit along with the React plug-in simplifies setup by running an npm command.
- Create React App was a popular option for building SPAs with React but is no longer recommended due to being bloated, slower, and unsupported.
- Vit is a newer solution that is faster, offers a better developer experience, and is built on ES Build, a fast JavaScript bundler.
- Vit includes a built-in development server with hot module replacement, making it ideal for building SPAs with React and other frameworks.
- The crash course aims to teach React fundamentals while creating a React jobs website with mock backend data using JSON Server.
- The project includes components like a hero component, navbar, job listings, and CRUD functionality without authentication.
- The project uses a Tailwind theme for styling, with source folders containing components and React files.
- Necessary tools include Node.js for Vit setup, React Developer Tools for component inspection, and React's official website for documentation.
- Setting up Tailwind involves installing Tailwind, PostCSS, and Autoprefixer, initializing Tailwind and PostCSS, and configuring Tailwind classes in the project.
- JSX syntax in React components allows for dynamic rendering, with the rule of returning a single parent element in each component.
31:16
"Essential JSX Tips for React Components"
- To avoid errors, wrap a div and a paragraph in a single element.
- Use a fragment, an empty HTML tag, to avoid an actual div if not desired.
- In JSX, dynamic variables can be inserted within curly braces.
- Expressions and variables can be utilized within curly braces in JSX.
- Loops can be implemented to render lists in JSX.
- When creating a list in JSX, ensure each element has a unique key prop.
- Conditionals can be used in JSX, but if statements must be avoided.
- Inline styles can be added in JSX using double curly braces.
- Styles can be stored in a variable and applied in JSX.
- Components can be created in separate files and imported into the main component in React.
47:18
Dynamic Content Styling with React Components
- To display content as title and subtitle, use curly braces and props.
- Destructure props to access title and subtitle directly.
- Default props can be set for title and subtitle.
- Wrapper components like cards can be created to encapsulate content.
- Create a separate component, like home cards, to hold multiple cards.
- Use the card component to style content with background colors and shadows.
- Props can be used to change content and styling dynamically.
- Data can be fetched from a JSON file and displayed using the map method.
- Create a job listing component to display individual job details.
- Each job listing should have a unique key, like the job ID, for proper rendering.
01:04:54
Efficiently Organizing UI Components in React
- In the home cards, there are two card components.
- In job listings, there are six job listing components.
- The job data is organized within the props, making the UI neat and manageable.
- Using front-end frameworks like React helps in organizing UI components efficiently.
- On the homepage, only three job listings are desired to be displayed.
- To achieve this, a recent jobs constant is created by slicing the jobs array to display only the first three.
- A "View All Jobs" button is placed in its own component for better organization.
- The useState hook is introduced to manage component state, allowing toggling of job descriptions.
- A button is added to toggle between showing a short or full job description based on state.
- React Icons package is installed to incorporate icons like the map marker in components.
01:21:50
"Creating Routes and Pages in React"
- Creating routes in React involves defining paths and elements.
- Routes can be set to display specific elements based on the path provided.
- Pages in React are components that can be organized into separate folders like "Pages."
- A "Layout" component can be created to display elements consistently across multiple pages.
- The "Outlet" component in a layout displays the content of the current page.
- Using the "Link" component in React Router Dom prevents full page refreshes when navigating between pages.
- Custom 404 pages can be created to handle non-existent routes.
- The "NavLink" component can be used to style active links in the navigation bar.
- Components like "Job Listings" can be reused across different pages by passing props.
- Dynamic styling for active links can be achieved by defining a function to handle class names.
01:41:37
Dynamic Job Listings with Json Server Integration
- The variable is set to false by default, then changed to "job listings" instead of "recent jobs."
- Depending on whether it is the homepage, the variable is set to either "job slice" or "just jobs."
- The list is created with "job listings" instead of "recent jobs," displaying all six on the homepage.
- The homepage is adjusted by passing in the prop "is home" set to true, showing only three jobs.
- The title is dynamically changed to "recent jobs" or "browse jobs" based on whether it's the homepage.
- Json server is introduced as a tool to create a mock API for fetching data from a backend.
- The Json file is modified to contain an object with a "jobs" array for the server to function.
- Json server is installed as a Dev dependency and configured to run on a separate port from the React app.
- Data fetching from the backend is implemented in the "job listings" component using useEffect and useState hooks.
- A spinner component is created using react-spinners to display loading while fetching data, centered using CSS overrides.
01:59:27
Efficient Data Fetching Techniques in React
- To replace Fetch with the API URL, update the jobs page with six and the homepage with three.
- Add a proxy to avoid changing the Local Host 8000 when deploying the project.
- In the V config file, under the server object, add a proxy for SL API to target HTTP Local Host Port 8000.
- Use rewrite to replace SL API with nothing, ensuring SL API slj jobs hits Local Host SL jobs.
- Data fetching using useEffect involves rendering while fetching, with a fallback UI like a spinner.
- React Suspense allows rendering while fetching data, with a fallback UI like a spinner.
- React Query and SWR are third-party libraries for easier data fetching than the base method.
- React 19 introduces the new use hook for data fetching, offering different ways to achieve the same result.
- Create a single job page using a data loader from React Router, fetching data without useEffect and exporting it for use in other components.
- Update the job page HTML file with dynamic data and replace hardcoded content with dynamic data.
02:18:11
"Job Listing Code Updates and Enhancements"
- Change margin right to 2 for the arrow in the code.
- Update the link to "link" and change it to "2" and "/jobs" for the link.
- Replace hardcoded "senior react developer" with data from the job object.
- Modify "full time" to "job.type" and "job.title" for the job title.
- Introduce the "FA map marker" icon for the frontend engineer.
- Adjust "Boston Mass" to "job.location" and "job.description" for the description and salary.
- Utilize the company object for company information like name, description, email, and phone.
- Create links for editing and deleting job listings.
- Establish the "add job page" under Pages and set the route to "add-job".
- Implement form fields with corresponding state management using "useState" for each field.
02:38:57
"Creating Full CRUD App with React"
- Passing a function as a value to add job submit and calling it in the page.
- Confusion regarding passing functions up or down in the component.
- Function add job being triggered when firing off in the component or page.
- Making a post request to add a job by using fetch with specific parameters.
- Successfully adding a job and redirecting to the job page.
- Implementing a delete function by passing it as a prop and confirming deletion.
- Using react-toastify for notifications on successful job addition and deletion.
- Setting up an edit job page and populating a form with existing job data.
- Updating job data by submitting the form with a put request.
- Completing a full CRUD application with the ability to add, delete, and edit jobs.
03:01:39
"Essential React Tutorial and Deployment Guide"
- Covered topics in the tutorial include components, props, State, React Router, data loaders, useEffect, useState, and various hooks in React.
- To deploy a project, run "npm run build" to generate a production version of the site, creating a "dist" folder. Use "npm run preview" to view the production build, which can be deployed after setting up an API with Express or similar tools for real-world projects.

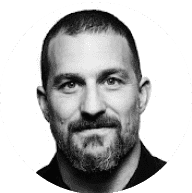
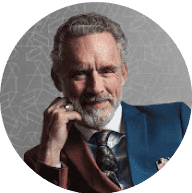
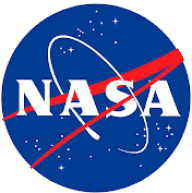
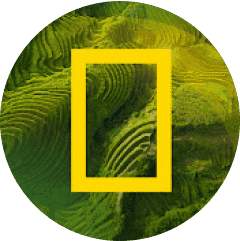