How to Use Environment Variables with a dotenv(.env) File for Node.js | Node Config with dotenv
ProgrammingKnowledge・2 minutes read
Environment variables in Node.js allow developers to store and manage sensitive information outside the codebase, facilitating easier configuration for different deployment environments. To set up these variables, developers can use the `dotenv` package to load values from a `.env` file and implement error handling to ensure that essential credentials are defined for the application to run properly.
Insights
- Environment variables in Node.js are essential for keeping sensitive information secure and enabling easy configuration changes across different deployment environments. By storing data like API keys and database URLs outside the codebase, developers can manage configurations without altering the actual code, enhancing security and flexibility.
- Setting up environment variables involves creating a project directory, initializing it with npm, and using the `dotenv` package to load variables from a `.env` file into the application. This process includes defining essential variables, implementing error handling for missing values, and following best practices, such as creating separate `.env` files for different environments and ensuring sensitive data is not included in version control.
Get key ideas from YouTube videos. It’s free
Recent questions
What are environment variables used for?
Environment variables are dynamic values that store sensitive information outside the codebase, such as API keys, database URLs, and passwords. This separation allows for easier configuration changes without modifying the actual code, making it particularly useful for applications that need to run in different environments, like development, testing, and production. By using environment variables, developers can ensure that sensitive data is not hardcoded into the application, which enhances security and flexibility. This practice also simplifies the deployment process, as different configurations can be easily managed without altering the source code.
How do I create a project in Node.js?
To create a project in Node.js, start by opening your terminal and navigating to your desired directory, such as the desktop, using the command `cd desktop`. Next, create a new project directory with `mkdir env_demo` and enter it by executing `cd env_demo`. Once inside the project directory, initialize your Node.js project by running `npm init -y`, which will generate a `package.json` file. This file is essential as it contains metadata about your project and its dependencies. After this setup, you can proceed to install any necessary packages and start developing your application.
How do I use the dotenv package?
The `dotenv` package is used in Node.js applications to manage environment variables conveniently. To use it, first install the package by running `npm install dotenv` in your project directory. This package allows you to load environment variables from a `.env` file into the `process.env` object, making them accessible throughout your application. After installation, create a `.env` file in your project root and add your sensitive information, such as `PORT=3000` and `API_KEY=your_api_key`. In your main JavaScript file, typically `index.js`, require the `dotenv` package by adding `require('dotenv').config()`. This line will load the variables defined in your `.env` file, allowing you to use them in your code securely.
Why should I handle missing environment variables?
Handling missing environment variables is crucial for maintaining the stability and security of your application. If an essential variable, such as an API key or database URL, is not defined, it can lead to runtime errors or unexpected behavior. To prevent this, you should implement error handling by checking if the required environment variables are set. For instance, you can check if `process.env.API_KEY` is defined and throw an error if it is not, using `throw new Error('Missing API Key')`. This proactive approach ensures that your application does not run without critical credentials, thereby avoiding potential failures and making debugging easier.
How can I manage different environments in Node.js?
Managing different environments in Node.js can be achieved by creating multiple `.env` files tailored for each environment, such as `.env.development`, `.env.testing`, and `.env.production`. This allows you to specify different configurations for development, testing, and production setups. To load the appropriate environment file based on the current environment, you can modify your code to specify the path in the `require('dotenv').config({ path: '.env.production' })` line, for example. Additionally, it is best practice to use a `.gitignore` file to exclude `.env` files from version control, ensuring that sensitive data is not exposed. Validating critical variables at application startup further enhances security and reliability, allowing for a smoother deployment process.
Related videos
Felipe Rocha • dicasparadevs
Curso de Node.js Para Completos Iniciantes
Rocketseat
COMEÇANDO COM NODE.JS EM 2022
Rocketseat
Como sair do ZERO em Node.js em apenas UMA aula
Dave Gray
Next.js Role-Based User Authorization & Access Control | Next Auth Protected Routes
Marko
A Day in The Life of a Software Engineer (ep. 45)
Summary
00:00
Managing Environment Variables in Node.js
- Environment variables in Node.js are dynamic values that store sensitive information such as API keys, database URLs, and passwords outside the codebase, allowing for easier configuration changes without modifying the code, especially useful for different deployment environments.
- To set up environment variables, open the terminal and navigate to the desktop using `cd desktop`, then create a project directory with `mkdir env_demo` and enter it using `cd env_demo`. Initialize the project with `npm init -y` to create a `package.json` file.
- Install the `dotenv` package by running `npm install dotenv`, which loads environment variables from a `.env` file into the `process.env` object. Create a `.env` file and add sensitive information such as `PORT=3000`, `API_KEY=your_api_key`, and `DB_URL=mongodb://localhost:27017/my_database`.
- In the `index.js` file, require the `dotenv` package with `require('dotenv').config()` to access the environment variables. Use `const PORT = process.env.PORT || 3000`, `const DB_URL = process.env.DB_URL`, and `const API_KEY = process.env.API_KEY` to assign these variables, ensuring the names match those in the `.env` file.
- Implement error handling for missing environment variables by checking if `process.env.API_KEY` is defined. If not, throw an error with `throw new Error('Missing API Key')` to prevent the application from running without critical credentials.
- For managing different environments, create multiple `.env` files (e.g., `.env.production`) for development, testing, and production configurations. Modify the code to load the appropriate file based on the current environment by specifying the path in `require('dotenv').config({ path: '.env.production' })`, and follow best practices such as not hardcoding sensitive data, using `.gitignore` to exclude `.env` files from version control, and validating critical variables at application startup.

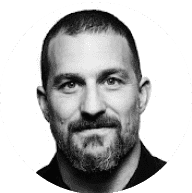
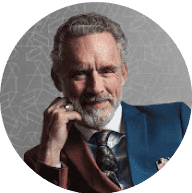
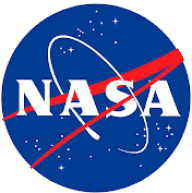
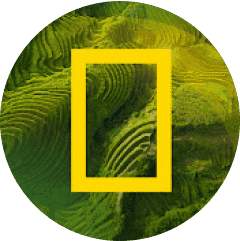