Realtime Chat App in React Native and AWS (Backend) 🔴
notJust․dev・6 minutes read
The text discusses building a real-time chat application integrated with an AWS Amplify backend, emphasizing the importance of actively building along with the live stream. It covers setting up authentication, implementing a GraphQL API, managing users, chat rooms, messages, and user interaction within the application.
Insights
- Setting up AWS Amplify locally involves detailed steps like signing up for an AWS account, configuring Amplify, and installing necessary libraries, simplifying backend development for chat applications.
- Utilizing Amplify for authentication streamlines the process by providing easy-to-use UI elements for sign up, login, and password recovery, enhancing user experience and security.
- Integrating GraphQL API with AWS Amplify enables seamless user registration, database linkage, and chat room creation, offering a robust foundation for real-time chat application development and user interaction.
Get key ideas from YouTube videos. It’s free
Recent questions
What is AWS Amplify?
AWS Amplify is a tool that combines various AWS services for backend development, providing easy setup and integration for applications. It simplifies tasks like setting up authentication, configuring APIs, and managing backend resources efficiently.
How can I set up authentication with AWS Amplify?
To set up authentication with AWS Amplify, start by signing up for an AWS account and configuring Amplify locally. Install necessary libraries, configure them in the app, and commit changes to Git. Add authentication by choosing a sign-in method like username, configure basic settings, and customize authentication flows easily.
What is the importance of using GraphQL with AWS Amplify?
GraphQL is crucial for interacting with the API in AWS Amplify, enabling efficient querying and mutation operations for user data. By implementing GraphQL, developers can easily fetch user information, create new users, and manage database interactions seamlessly within the application.
How can I create chat rooms in an application using AWS Amplify?
To create chat rooms in an application with AWS Amplify, define a new model "chat room" in the backend API schema. Establish relationships between users and chat rooms by adding a "chat room user" model. Implement queries and mutations to support creating, updating, and deleting chat rooms, ensuring seamless user interactions within the chat application.
What is the process of sending messages in a chatroom with AWS Amplify?
Sending messages in a chatroom with AWS Amplify involves creating a new type called "message" with specific fields. Query messages based on the chatroom ID and sort them by creation date. Retrieve the user ID from the authenticated user to send messages, call the "create message" GraphQL operation, and display messages in the chatroom for real-time communication.
Related videos
Summary
00:00
"Building Real-Time Chat App with AWS"
- Building a real-time chat application integrated with an AWS Amplify backend with a GraphQL API and authentication services.
- Previous week's progress included creating a WhatsApp clone with UI elements like messages and a flat list.
- Encouragement to subscribe to the channel for similar content and join the Discord server for sharing builds and learning.
- Emphasizing the importance of actively building alongside the live stream to truly grasp concepts.
- Demonstrating the existing chat application with features like a list of chats, chat rooms, and a contacts list.
- Plans for the current session involve integrating the application with AWS Amplify, setting up authentication, and implementing a GraphQL API.
- Celebrating reaching 1,000 subscribers and expressing excitement for future content.
- Introduction to AWS Amplify as a tool combining various AWS services for backend development.
- Detailed steps for setting up AWS Amplify locally, including signing up for an AWS account and configuring Amplify.
- Installing necessary libraries for Amplify, configuring it in the app, and committing changes to Git.
- Adding authentication to the application through Amplify, choosing username as the sign-in method, and configuring basic settings.
25:15
"Amplify: Simplifying Authentication Setup in Apps"
- Amplify provides easy-to-use UI elements for authentication screens like sign up, login, and forget password.
- By importing the authenticator and wrapping the application, all authentication screens are set up with just two lines of code.
- Running "amplify console" opens the AWS console to view the application in the cloud.
- With the authenticator code, the app is protected by authorization, requiring sign up and login.
- A confirmation code is sent to the email for verification during sign up.
- The application is logged in successfully after entering the confirmation code.
- Amplify allows customization of authentication flows and provides methods for sign in and sign up.
- Setting up analytics can resolve promise rejection warnings in the application.
- Implementing a sign-out button using the sign-out function from the authentication service allows easy log out.
- The next step involves committing the changes to Git, marking the completion of the authentication setup.
52:09
"Async function for one-time mount operation"
- To ensure a function runs only once when mounted, declare a sync function like 'patch user' or 'manage user' and call it immediately after declaration.
- The function must be async due to limitations with 'useEffect', which requires a non-async function.
- The first step involves getting the authenticated user, followed by fetching the user from the backend using the user ID from authentication.
- If no user exists in the database with the ID, create one to complete the flow.
- In 'useEffect', the dependencies array specifies when the function should run; an empty array ensures it runs only on mount.
- Implementing the first step involves awaiting 'user info' and importing 'authentication' from AWS Amplify.
- 'User info' may be null if the user is not authenticated, so a check is necessary before proceeding.
- Fetch the user from the backend using the user ID obtained from 'user info' to link the user with the database.
- Utilize GraphQL queries and mutations, such as 'get user' and 'create user', to interact with the API for user operations.
- The process involves querying for existing users and creating new ones if none are found, ensuring a seamless user registration and database linkage process.
01:22:55
"iOS App Customization and User Management"
- To remove the default back button on iOS applications, send a null value to the header back button.
- To display all users in the contact list, use a useEffect function to fetch users from the backend API.
- Use the list users GraphQL operation from Amplify to retrieve user data from the server.
- Save the received user data in a state variable using useState to display it in the component.
- Manually add users through the console to test and display them in the contact list.
- Create a new model "chat room" in the backend API schema to support chat rooms with multiple users.
- Establish a many-to-many relationship between users and chat rooms by adding a separate model "chat room user" to link them.
- Define connections between users, chat rooms, and chat room users to enable querying information about users and chat rooms.
- Implement the creation of chat rooms and chat room users when starting a chat with another user.
- Update queries and mutations in the code to support creating, retrieving, updating, and deleting chat rooms and users.
01:52:30
"Creating Chat Rooms with AWS Amplify"
- Import necessary libraries and APIs like AWS Amplify and GraphQL
- Create mutations for creating a chat room and adding users to it
- Implement steps to set up a chat room: create a new chat room, add a user, and add an authenticated user
- Utilize GraphQL operations to create a new chat room with auto-generated IDs
- Handle errors and console log messages for successful chat room creation
- Add users to the chat room using GraphQL operations with user and chat room IDs
- Query user data to display chat rooms and users in the chat room
- Modify GraphQL queries to include necessary data like chat room users and their details
- Parse and display chat room data including users and messages
- Define state for chat rooms and update it with data from the GraphQL query to display chat rooms on the screen
02:23:40
Mapping Chatroom Users with AWS Amplify GraphQL
- The chatroom users need to be mapped due to the complexity of the data structure.
- Working with Apollo and GraphQL can be challenging, especially for advanced features.
- Firebase is beginner-friendly, while AWS Amplify is more suitable for production-ready applications.
- Implementing messages in the chatroom involves creating a new type called "message" with specific fields.
- A secondary key for sorting messages by chatroom and creation date is crucial.
- The process involves querying messages based on the chatroom ID and sorting by creation date.
- The backend is built using AWS Amplify with a GraphQL API.
- The user ID is essential for sending messages and is retrieved from the current authenticated user.
- The creation of messages in the chatroom involves calling the "create message" GraphQL operation.
- The chatroom ID is passed as a parameter to the input box component for sending messages.
03:02:10
Debugging, messaging, chatrooms, and future plans
- Open the debugger to view "hello world" messages
- Create a list of messages, updating the page with "hello world"
- Create a chatroom, messages, and users for notifications
- Discuss using Amplify or creating a GraphQL server
- Implement a query for messages by chatroom
- Fetch data from the API for the chatroom screen
- Resolve a design bug with the keyboard
- Plan real-time functionality with subscriptions
- Discuss future plans for the application and user interaction

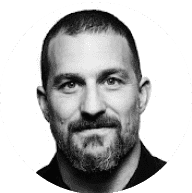
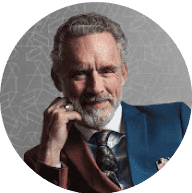
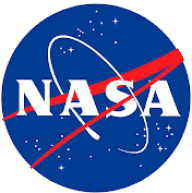
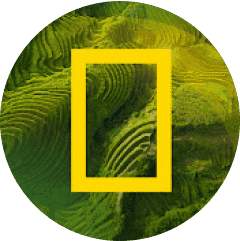