Java Programming for Beginners – Full Course
freeCodeCamp.org・145 minutes read
The text introduces Java basics using Replit, an online IDE, with detailed instructions on programming concepts like variables, operators, strings, arrays, loops, ArrayLists, hash maps, and object-oriented programming. By emphasizing practice, understanding classes, objects, properties, methods, constructors, and inheritance, learners are encouraged to focus on mastering foundational concepts before tackling advanced topics like method overloading, overriding, and abstract methods in Java.
Insights
- Replit is an online IDE utilized by Farhan Hassan Chodri to teach Java basics, enabling programming in various languages through a web browser.
- Java source files end with .java, compiled byte codes with .class; Replit permits viewing compiled byte codes, crucial for understanding program execution.
- Java introduces primitive data types for numbers, emphasizing the significance of type conversion, both implicit and explicit, ensuring data integrity.
- Arithmetic operators in Java, such as addition, subtraction, multiplication, division, and modulus, are fundamental for mathematical operations in programming.
- Understanding Java strings is vital, including creating, manipulating, and comparing strings, highlighting key methods like equals, equalsIgnoreCase, replace, and contains.
Get key ideas from YouTube videos. It’s free
Recent questions
What is the main method in Java?
Entry point to a Java program.
What are assignment operators in Java?
Simplify adding values to variables.
How are strings created in Java?
Using literal way or new keyword.
What are conditional statements in Java?
Used for logical decision-making.
What are the different types of loops in Java?
For, while, do-while loops.
Related videos
Apna College
Java OOPs in One Shot | Object Oriented Programming | Java Language | Placement Course
Rocketseat
Como sair do ZERO em JAVA em 1h - com @kipperdev
Error Makes Clever
Python Tutorial - Python Full Course for Beginners in Tamil
Apna College
Functions & Methods | Java Complete Placement Course | Lecture 7
Sir Tarun Rupani
Library Classes in Java | Computer Application ICSE Class 10 | @sirtarunrupani
Summary
00:00
"Java Basics with Replit: A Primer"
- Farhan Hassan Chodri, an experienced software developer at Free Code Camp, teaches Java basics using Replit, an online IDE.
- Replit allows writing various programming languages in a web browser and was crucial in making the course possible.
- To start, go to www.replit.com, create an account or log in, and create a new Java Replit with a descriptive name.
- The default Java Replit includes the famous "Hello World" program, which you can run by clicking the run button.
- Java source files end with .java, and compiled byte codes end with .class; Replit allows viewing compiled byte codes.
- The main method in Java serves as the entry point to a program, following specific criteria set by the JVM.
- Variables in Java must be declared and defined, with specific rules for initialization and usage.
- Naming variables in Java should follow certain conventions, avoiding reserved keywords and excessive use of symbols.
- Java has primitive data types for numbers, including bytes, shorts, integers, longs, doubles, floats, booleans, and characters.
- Type conversion in Java can be implicit or explicit, with the cast operator used for explicit conversions, ensuring data integrity.
21:32
Java Arithmetic and Logical Operators Explained
- Arithmetic operators in Java include addition, subtraction, multiplication, division, and remainder.
- Code example with two integer numbers, 12 and 6, showcases addition resulting in 18, subtraction in 6, multiplication in 72, and division in 2.
- Subtraction outcome changes based on operand order; 12 - 6 = 6, while 6 - 12 = -6.
- Division of integers yields integer results, changing to double operands provides decimal results.
- Modulus operation calculates the remainder after division; 12 % 6 = 0, 12 % 8 = 4.
- Assignment operators, like +=, simplify adding values to variables; e.g., number += 5 updates number to 17.
- Utilizing assignment operators with arithmetic operators streamlines operations; e.g., number -= 6 reduces number to 6.
- Relational operators compare values, returning true or false based on conditions like equality, inequality, greater than, less than, greater than or equal to, and less than or equal to.
- Logical operators like && (and) and || (or) combine conditions for decision-making; && requires both sides to be true, while || needs at least one side to be true.
- Unary operators, including increment (++) and decrement (--), modify values by one; ++ increases, -- decreases.
43:58
Exploring Java Strings and Operators
- Incrementing a value by 1 in the EU from 55 to 56 is explored.
- The value does not increment as expected when using the increment operator.
- Making a copy of a line and rerunning the program shows a change in value.
- The increment operator on line 5 does not change the value, but on line 6, it does.
- Compiler behavior is explained, showing how values are processed.
- The decrement operator is discussed, showing how values change.
- Different ways of writing the increment and decrement operators are demonstrated.
- The character type in Java is introduced as a primitive type.
- Strings in Java are explained as a way to store multiple characters.
- Two methods of declaring a new string in Java are detailed.
- The new keyword in Java is used to create new objects from classes.
- The difference between creating strings using the literal way and the new keyword is explained.
- The concept of a string pool in Java is introduced.
- An example is provided to demonstrate the difference between creating strings using the literal way and the new keyword.
- The importance of choosing the right technique for creating strings is emphasized.
- Essential methods for manipulating strings in Java are introduced.
- The process of formatting strings in Java is explained.
- Format specifiers for strings, integers, floating-point numbers, characters, and booleans are detailed.
- An example is provided to demonstrate formatting strings using format specifiers.
- The length and isEmpty methods for strings in Java are explained.
- Converting strings to uppercase and lowercase in Java is demonstrated.
01:09:51
Java String Methods and User Input Handling
- Uppercase method in Java doesn't alter the original string; adding a println statement confirms this.
- Lowercase method in Java converts the entire string to lowercase.
- Two ways to declare new strings in Java: literal way and using the new keyword.
- Creating strings using the new keyword results in separate objects; equality operator checks object identity.
- To compare strings in Java, use the equals method instead of the equality operator.
- For case-insensitive string comparison, utilize the equalsIgnoreCase method.
- The replace method in Java replaces a substring within a string without altering the original string.
- To check if a string contains a specific word or substring, use the contains method in Java.
- Taking user input in Java involves creating a Scanner object and using methods like nextLine and nextInt.
- Handling input buffer issues when mixing nextInt and nextLine calls by adding an extra nextLine call to clear the buffer.
01:34:43
"Introduction to Conditional Statements in Programming"
- The text introduces the concept of conditional statements in programming.
- It explains the importance of conditional statements in making logical decisions based on conditions.
- The text mentions the need for a scanner to take user input.
- It details the process of taking input from the user for two numbers using the scanner.
- It emphasizes the use of if statements in Java to check conditions.
- The text provides an example of using if statements to perform addition based on user input.
- It highlights the importance of using else statements for situations where if conditions are not met.
- The text introduces the concept of nested if blocks for more complex conditions.
- It explains the implementation of subtraction, multiplication, and division operations using if-else statements.
- The text introduces switch-case statements as an alternative to if-else statements for branching code based on conditions.
02:01:43
Understanding Arrays in Java Programming
- Arrays in Java have a length of 5, starting from index 0 and ending at 4.
- Attempting to access an index beyond the array's length results in an "index out of bounds" exception.
- Arrays can be declared and defined in a single line by using curly braces to set values.
- Values in an array can be replaced by specifying the index and the new value.
- The length of an array is inferred from the number of values defined during declaration.
- Strings arrays in Java are reference types with useful methods like determining the array length.
- The arrays class includes a sort method to arrange arrays in order.
- The sort method can be used within a specific range of the array by defining starting and ending indices.
- The binary search method in arrays works only on sorted arrays to find specific values.
- Arrays can be filled with a specific value using the fill method, with options for starting and ending indices.
02:26:37
Java Loops: Equality, Arrays, and Iteration
- The equality operator is used to compare numbers for equality.
- When comparing arrays for equality, the `arrays.equals` method is used.
- There is no `equalsIgnoreCase` method for arrays like there is for strings.
- Loops are used to repeat instructions in programming projects.
- There are four types of loops in Java, starting with the for loop.
- A simple for loop in Java can print numbers from 1 to 10.
- The for loop has four parts: initialization, condition, loop body, and update.
- A for loop can also be used to iterate over an array and print its elements.
- The sum of numbers from 1 to 10 can be calculated using a for loop.
- Nested for loops can be used to print multiplication tables and perform more complex operations.
02:50:15
Java ArrayList: Basics, Methods, and Manipulation
- While loops and do-while loops have different structures and execution orders.
- In a do-while loop, the loop body is executed before the condition is checked.
- To use an ArrayList in Java, import the ArrayList class and specify the data type.
- The Integer class is a wrapper class for the primitive type int, necessary for ArrayLists.
- Adding items to an ArrayList is done using the add method.
- Printing an ArrayList requires using the toString method.
- Removing items from an ArrayList can be done by index or value.
- ArrayLists can store various data types and objects.
- Sorting and reversing an ArrayList can be achieved using the sort and reverse methods.
- Additional methods like size, contains, isEmpty, and set are available for ArrayList manipulation.
03:15:56
Managing Hash Map Operations in Java
- The hash map prints scores for English (95), sociology (85), and math (75).
- Items in the hash map are not sorted, leading to random order display.
- To print a single value from the hash map, use the name of the hash map followed by ".get(key)".
- The "putIfAbsent" method checks if a value exists in the hash map before adding it.
- The "replace" method updates a value in the hash map.
- Use "getOrDefault" to retrieve a value with a default if the key doesn't exist.
- To clear a hash map, use "hashMap.clear()".
- Check the number of elements in a hash map with "hashMap.size()".
- Remove an item from the hash map using "hashMap.remove(key)".
- Use "containsKey(key)" or "containsValue(value)" to check if an item exists in the hash map.
03:40:55
"Creating and Displaying Book Objects"
- Create a new file named "book.java" in the files menu
- Declare a new class named "Book" with public fields for title and author
- Initialize a new book object in the main class with title "Carmilla" and author "Shelton"
- Implement a method in the user class to borrow books, adding the borrowed book to a list
- Define a custom toString method in the book class to display book details
- Encounter issues with random values due to missing toString method in the custom book class
- Resolve the issue by implementing a custom toString method in the book class
- Introduce the concept of Constructors to initialize class properties with default values
- Create a Constructor in the user class to set name and birthday during object creation
- Implement private properties and getter methods for name and birthday in the user class
- Apply abstraction by hiding complexities of class properties behind getter methods
- Make properties private in the book class and implement getter methods for title and author
- Update book creation process to use Constructor and getter methods for title and author
- Make the books list private in the user class and implement a getter method for borrowed books
- Implement inheritance by creating an audio book class extending the book class
- Add a runtime property to the audio book class and update Constructor to include necessary properties
- Create a new audio book object with title "Dracula" and runtime of 30,000 minutes
- Display the details of the audio book using the toString method in the main class
04:07:11
Creating ebook objects in Java programming.
- Create a new class named "ebook" that extends a previous class, adding private string variables for format and page count, then instantiate an ebook object with specific details like author, page count, and format (e.g., "Carry on Jeeves" by PG Wodehouse, 280 pages, PDF format), demonstrating the creation of different types of books under a parent class in object-oriented programming. The course covers classes, objects, properties, methods, constructor methods, and inheritance, encouraging thorough understanding and practice before delving into more advanced concepts like method overloading, overriding, and abstract methods. The advice is to focus on mastering the current concepts, practicing, and gradually exploring complex ideas through practical application in Java programming.

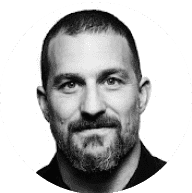
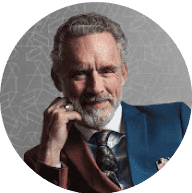
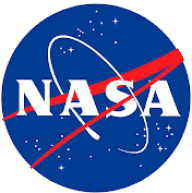
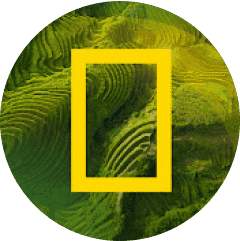