Emulating a CPU in C++ (6502)
Dave Poo・2 minutes read
Emulating a CPU like the 6502 involves understanding its architecture, memory structure, and operational principles, requiring proper initialization and execution of instructions. The process includes handling various addressing modes, clock cycles, and implementing specific functions to execute programs successfully while emulating the actions of a C64 computer.
Insights
- Emulating a CPU like the 6502 involves replicating key components such as memory, registers, and execution cycles, with specific initialization steps required for proper functioning.
- The 6502 processor, though outdated, shares fundamental principles with modern processors, showcasing concepts like memory-mapped I/O, addressing modes, and flag registers, making it a valuable tool for understanding CPU architecture and operation.
Get key ideas from YouTube videos. It’s free
Recent questions
How does memory-mapped I/O work?
Memory-mapped I/O connects hardware devices to specific memory regions. This concept allows the CPU to communicate with peripherals by reading from or writing to memory addresses associated with those devices. By treating hardware registers as if they were memory locations, the CPU can easily interact with external components without the need for specialized instructions, simplifying the overall system design.
What are the key registers in a 6502 CPU?
The 6502 CPU features three 8-bit registers: the program counter, stack pointer, and two index registers (X and Y). These registers play crucial roles in executing instructions, managing memory access, and performing calculations within the processor. The program counter keeps track of the memory address of the next instruction to be executed, while the stack pointer points to the current location in the stack memory. The index registers are versatile for various operations, such as array manipulation and data copying.
How are instructions executed in a 6502 CPU?
Instructions in a 6502 CPU are executed by fetching the next instruction, decoding its operation, and then performing the necessary actions. Each instruction has specific addressing modes and cycle requirements that dictate how it interacts with memory and registers. The CPU fetches the instruction byte, interprets the opcode, and executes the operation based on the addressing mode specified. By following a precise sequence of steps, the CPU can accurately carry out the desired instruction, advancing the program counter and updating registers as needed.
What is the significance of the zero page in a 6502 CPU?
The zero page in a 6502 CPU refers to the first 256 bytes of memory that are directly addressable with shorter instructions, allowing for faster access compared to other memory locations. This region is crucial for optimizing performance in programs that require frequent data manipulation or quick memory access. By utilizing the zero page effectively, programmers can streamline their code and improve the overall efficiency of the CPU's operations.
How does the reset process initialize a 6502 CPU?
The reset process in a 6502 CPU involves setting key values like the program counter, stack pointer, and registers to predefined states to prepare the processor for execution. During initialization, memory is configured, and the CPU is placed in a known state to ensure proper functionality. By establishing a consistent starting point, the reset routine enables the CPU to execute instructions correctly and interact with memory and peripherals as intended. This initialization step is essential for emulating the behavior of a 6502 CPU accurately.
Related videos
Summary
00:00
Understanding 6502 CPU Emulation and Operation
- Emulating a CPU to understand its workings involves writing an emulator for a CPU, like the 6502, a simple 8-bit processor.
- The 6502 processor, despite being old, shares operational principles with modern processors, such as memory, code execution, and registers.
- The 6502 CPU has a 16-bit address bus, allowing access to 64 kilobytes of memory, with a little-endian processor design.
- The CPU features a "zero page," the first 256 bytes of memory, crucial for faster access due to historical memory limitations.
- The second memory page is dedicated to stack memory, with reserved memory addresses for various functions, including the reset vector.
- Memory-mapped I/O was used to connect hardware devices to specific memory regions, a concept seen in the 6502 processor.
- The 6502 CPU has three 8-bit registers: the program counter, stack pointer, and two index registers (X and Y).
- The processor status register contains flags like carry, zero, interrupt disable, decimal mode, break command, overflow, and negative.
- Emulating the 6502 CPU involves initializing key values like the program counter, stack pointer, and registers during the reset process.
- The reset routine initializes memory and sets the CPU into a state ready for execution, with memory struct and CPU struct interaction essential for proper functioning.
14:21
Emulating C64 CPU for instruction execution.
- The process involves emulating the CPU and the actions of a C64 computer.
- Initialization includes setting up the CPU, memory, and executing a reset.
- To execute instructions, a specific number of clock cycles need to be defined.
- Instructions like "load accumulator" have various addressing modes and cycle requirements.
- Each instruction in assembly language translates to machine code with specific byte counts.
- Execution involves passing the number of cycles to execute an instruction.
- Fetching the next instruction involves incrementing the program counter and fetching the byte from memory.
- The process of fetching an instruction requires decrementing the cycle count.
- A simple program is hard-coded into memory to test instruction execution.
- The program is set to execute a specific instruction with a defined cycle count.
30:44
"Emulating CPU Instructions: Clock Cycles and Flags"
- The value set into the A register is 42 after fetching it.
- Checking if A is zero and the negative flag is set, but neither condition is met.
- The CPU cycle is completed after executing two instructions.
- The program counter is advancing, potentially causing memory overflow.
- Writing an emulator for a CPU involves handling numerous instructions and addressing modes.
- Implementing instructions like loading from zero page requires specific steps and clock cycles.
- Reading a byte from zero page takes three clock cycles.
- Setting flags after executing an instruction can be extracted into a separate function.
- Implementing a jump to subroutine instruction involves fetching a 16-bit address and manipulating the stack.
- The jump to subroutine function takes six cycles and three bytes to execute.
47:47
"CPU Emulation: 699 Instructions, Random Access"
- The program starts with 699 instructions, resulting in 84 in the A register after running it.
- The program can jump around in memory, showcasing random access capabilities.
- Emulating a CPU involves understanding how it works, with the need for interfacing with other chips like a video chip for full functionality.

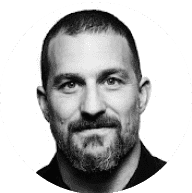
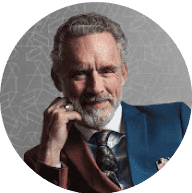
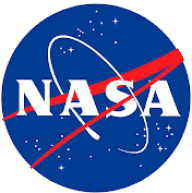
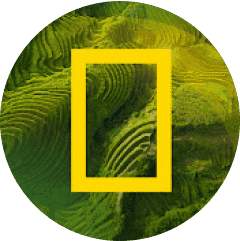