Complete Dynamic Programming Practice - Noob to Expert | Topic Stream 1
Colin Galen・2 minutes read
The text delves into dynamic programming, explaining recursive and iterative approaches to problem-solving with detailed examples and illustrations. It emphasizes the importance of defining states, transitions, and base cases for efficient computations.
Insights
- Dynamic programming is explained through the lens of solving the Fibonacci sequence problem, showcasing recursive and iterative approaches, as well as the optimization technique of memoization.
- The importance of defining states, transitions, and base cases in dynamic programming problem-solving is emphasized, preventing infinite computations and enabling efficient solutions.
- Various problem-solving scenarios, such as filling tiles or counting substrings, are tackled using dynamic programming techniques like recurrence relations and iterative optimizations, showcasing the versatility of this approach.
- Deriving formulas, counting derangements, and optimizing calculations through dynamic programming are explored, highlighting the complexity of mathematical concepts and the application of efficient methods to prevent overflow and ensure accuracy.
Get key ideas from YouTube videos. It’s free
Recent questions
What is dynamic programming?
A method for solving problems by breaking them down.
Related videos
Error Makes Clever
Python Tutorial - Python Full Course for Beginners in Tamil
Magnet Brains
Miscellaneous Exercise (Q1-Q18) - Integrals | Class 12 Maths Chapter 7 (2022-23)
Apna College
Java OOPs in One Shot | Object Oriented Programming | Java Language | Placement Course
Wolfram
Wolfram Physics Project: Working Session Tuesday, Aug. 4, 2020 [Empirical Physical Metamathematics]
Science and Fun Education
Sequences and Series One Shot Maths | Class 11 Maths Full NCERT Explanation by Ushank Sir
Summary
00:00
Optimizing Fibonacci: Memoization vs Iterative DP
- The speaker is setting up a stream, addressing potential audio issues due to lawn mowing outside.
- They greet a viewer named Crystal Violet and mention a tradition.
- The speaker discusses dynamic programming, starting with a simple problem like Fibonacci sequence.
- They explain the recursive approach to solving Fibonacci and its inefficiencies due to repeated calculations.
- Introducing memoization as a solution, they detail how storing previously computed values optimizes the process.
- The speaker illustrates memoization with a cache array and its impact on computational complexity.
- They transition to iterative dynamic programming, emphasizing a bottom-up approach to computing values.
- The iterative method involves a loop to calculate values in increasing order, storing them in an array.
- The speaker contrasts memoization (top-down) with iterative DP (bottom-up) and introduces a preferred method called push DP.
- Push DP involves values contributing to the calculation of subsequent values, offering a different perspective on dynamic programming implementation.
19:22
Dynamic Programming: Solving Problems with Recursion
- The text discusses the concept of dynamic programming and its application to problem-solving.
- The speaker explains the importance of defining the state and transitions in dynamic programming.
- Base cases, such as f(0) = 1 and f(1) = 1, are crucial in dynamic programming to prevent infinite computations.
- The problem at hand involves finding the number of ways to fill all three times n tiles with a specific shape.
- The speaker breaks down the problem into cases based on the placement of the shape in the top left corner.
- Through analysis, it is determined that there are only two viable ways to fill the tiles, leading to a recursive formula.
- The recurrence relation for the problem is f(n) = 2 * f(n-2), indicating the number of ways to fill the tiles based on previous computations.
- The speaker emphasizes the iterative approach to dynamic programming as an optimization technique.
- The discussion touches on the speaker's learning journey with dynamic programming, highlighting the importance of practice and examples.
- The overall focus remains on explaining the problem-solving process using dynamic programming for beginners.
38:00
Counting substrings with broken keyboard using arrays.
- Converting parentheses to brackets creates arrays, enabling a recurrence to compute f(n) using f(m-2) for f(n) and f(m).
- To compute f(m), previous values are needed, so f(n) is calculated after f(0) to f(n-1) in increasing order.
- Base cases are f(0) = 1 and f(1) = 0, as with zero rows there's one way and with one row, no way to fill tiles.
- The formula for f(n+1) is f(0) = 1, f(1) = 0, and for i from 2 to n, f(i) = 2 * f(i-2).
- Finding the state, necessary information, base cases, and recurrence is crucial in problem-solving.
- It's advisable to determine the recurrence first to establish the base cases effectively.
- Base cases are straightforward, involving specific values that can be easily computed.
- The code for the problem involves setting f(0) = 1, f(1) = 0, and f(2) = 2.
- The problem involves finding the number of substrings that can be typed using a broken keyboard with limited characters.
- A universal trick in counting problems is fixing a specific aspect, like the end character, to simplify calculations.
58:54
"Finding Substring Counts with Binary Numbers"
- Define f of i as the number of substrings consisting solely of ones that end at i.
- If a[i] equals zero, f of i is zero; if a[i] equals one, f of i is determined by the previous character's f value.
- The recurrence for f of i is the sum of substrings that can be extended plus one.
- The final answer is the sum of all f values over all possible ending points.
- For a string of length zero, there are no substrings, establishing the base case.
- To solve a character-based problem, define a function f of x to determine the answer.
- Represent the presence of vitamins A, B, and C in juices using binary numbers.
- Utilize bit mask DP to find the minimum cost to obtain all three vitamins.
- Introduce f of mask as the lowest cost to satisfy a specific mask.
- Implement push DP to consider transitions for each string and mask combination.
01:20:03
"Calculating Minimum Cost with String Masks"
- The cost of taking a string is the cost of string i plus 1, considering f of i mask.
- The base case is having zero strings, resulting in zero costs.
- A binary number is represented between zero and seven in an array of size eight.
- Initializing everything to infinity ensures no incentive to use certain states.
- Defining infinity as a large number like 10^17 helps in never reaching certain states.
- The answer is defined as infinity, and the cost of a string is calculated.
- The position in the mask is represented by an integer, with a specific notation for characters.
- The string mask is updated based on the position in the mask.
- Iterating through all possible values and masks to find the minimum cost for each case.
- The minimum cost is determined by considering all strings and having the full mask of a, b, and c.
01:44:35
"Optimal Assignment for Key Retrieval Problem"
- The interval for the suffix i plus k is the suffix i minus one or the prefix of i minus one.
- The current value equals zero if i is greater than zero.
- If i plus k is less than n, the curve plus equals the p value of i minus one.
- To solve a problem involving n people and keys in a straight line, each person must reach a key, take it, and then proceed to the office.
- The assignment of people to keys must be in increasing order to avoid crossed paths.
- The green assignment, where paths do not cross, is always better than other assignments.
- The DP state is defined as dp of position and last person assigned.
- The minimum cost to reach a position and use a certain number of people is calculated using push DP.
- The DP array is defined with n+1 positions and k+1 people, initialized to infinity except for dp[0][0].
- Transitions in the DP involve either taking a person or not, with the goal of reaching dp[k][n].
02:07:46
Counting Almost Identity Permutations with Constraints
- The text discusses a problem related to permutations, where an almost identity permutation is defined as one where at least n minus k indices have the property p i = i.
- The task is to count the number of almost identity permutations given n and k, with n up to a thousand and k up to four.
- An identity permutation is one where every number is equal to its index.
- An almost identity permutation allows for up to k elements to be out of order.
- The text delves into the process of determining the number of almost identity permutations by considering different values of x, representing the number of elements out of order.
- For x = 1, there are no possible permutations as changing one element leads to contradictions.
- For x = 2, the number of ways to pick two positions to put them out of order is calculated using the combination formula.
- When x = 3, the text explains the process of selecting three elements to be out of order, leading to cycles of length three in permutations.
- The discussion further extends to x = 4, where the text explores the concept of selecting four elements to be out of order in permutations.
- The text also briefly touches on the relevance of IQ or intelligence in competitive programming, highlighting its role in pattern recognition but emphasizing that it is not a limiting factor in skill development.
02:35:13
"Derangements, Factorials, and Efficient Calculations"
- The text discusses the concept of derangements and the formula for calculating n choose k.
- It explains the factorial function and cautions against computing factorials to avoid overflow.
- The text delves into the formula for n choose k to prevent overflow, emphasizing a more efficient method.
- It mentions the use of modulo to ensure that n choose k remains an integer.
- The text explores the concept of derangements and the use of dynamic programming to derive formulas.
- It discusses the process of counting derangements and the application of recursion or dynamic programming.
- The text mentions the complexity of the mathematical calculations and the challenges faced in understanding the formulas.
- It touches on the inclusion-exclusion principle and generating functions in the context of derangements.
- The text concludes with a summary of the process of counting derangements and the use of dynamic programming for calculations.
- It ends with a reflection on the complexity of the mathematical concepts discussed and a desire to return to more straightforward topics.
03:09:06
"Dynamic Programming for Font Size Sequences"
- The maximum speed desired is around a thousand, with t being a hundred and d less than or equal to 10.
- The stream will continue for a while, with the archive available later, including timestamps for easy navigation.
- The current value is at most 100, transitioning through at most 21 elements, possibly lasting over 30 minutes.
- The problems being tackled are not sorted by difficulty, with a to d being the easiest, while e to o are random.
- Facecam will be activated at 10,000 subs, with a special video planned for 5,000 subs.
- The dynamic programming problem involves finding a sequence of increasing font sizes with minimal cost.
- The approach involves considering whether an element starts a sequence or extends one, with a maximum length of three.
- The transition involves either starting a sequence or extending one, with the minimum cost calculated accordingly.
- The final answer is the minimum cost for a sequence ending at the last element, with a check for a valid sequence.
- The solution for problem h is refined, with a focus on the dynamic programming approach for optimal results.
03:39:57
Efficient Sequence Finding Algorithm with DP
- The task involves finding good sequences where each number divides the next number given n and k.
- To derive the state, build the dynamic programming (dp) on the position and the number.
- The complexity is manageable due to the limited number of multiples for each value, leading to a total complexity of O(kn log n).
- The process involves setting a virtual one at the beginning of a sequence, considering all values are at most n and divide one.

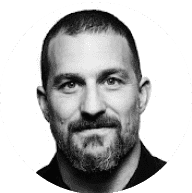
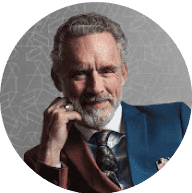
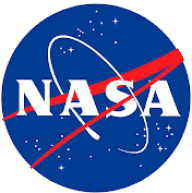
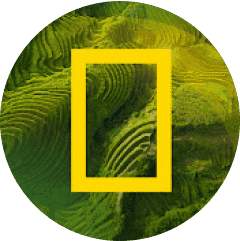