🔴 Build the Uber clone in React Native (Tutorial for Beginners)
notJust․dev・2 minutes read
The project involves building an Uber clone in React Native with AWS Amplify, featuring a home screen, search capabilities, and Uber trip options. Technologies used include React Native, Google APIs for maps and autocomplete, and learning points focus on setting up a React Native environment and managing navigation for the application.
Insights
- The project aims to create an Uber clone using React Native with AWS Amplify, incorporating features like map display, COVID messages, and trip options.
- The development process involves setting up a project structure, resolving errors, styling components, and integrating Google APIs for maps and autocomplete functionality.
- Key considerations include installing necessary libraries, configuring fonts, styling components, obtaining Google Places API keys, and implementing custom markers for map views in the application.
Get key ideas from YouTube videos. It’s free
Recent questions
How can I build an Uber clone?
To build an Uber clone, you will need to use React Native for the front end and AWS Amplify for the full-stack application. The application should include a home screen with a map displaying the user's location and a message related to COVID. Implement a search bar with Google autocomplete integration for address suggestions. Users should be able to select trip options like UberX, Comfort, and Excel, with displayed prices and a route map. Unique features like map directions, Google autocomplete, and a drawer navigator should be included. Utilize technologies like React Native, JavaScript, React functional components, and React hooks. Libraries such as React Navigation and Vector Icons will be necessary, along with Google APIs for maps and autocomplete. Ensure you have a set React Native environment, a Google developer account with billing enabled, and access to dummy data and assets before starting the project.
What are the key features of the Uber clone?
The Uber clone project in React Native will have a home screen with a map displaying the user's location and a message related to COVID. It will include a search bar with Google autocomplete integration for address suggestions. Users will be able to select trip options like UberX, Comfort, and Excel, with displayed prices and a route map. Unique features such as map directions, Google autocomplete, and a drawer navigator implementation will be incorporated. Technologies like React Native, JavaScript, React functional components, and React hooks will be used. Libraries like React Navigation and Vector Icons will be utilized, along with Google APIs for maps and autocomplete. The project will involve setting up a React Native project, rendering elements, creating reusable components, and managing navigation.
What are the prerequisites for building the Uber clone?
Before starting the Uber clone project, ensure you have a set React Native environment, a Google developer account with billing enabled, and access to dummy data and assets. These prerequisites are essential for setting up the project, rendering elements, creating reusable components, and managing navigation. Having a Google developer account with billing enabled is crucial for utilizing Google APIs for maps and autocomplete. Access to dummy data and assets will help in populating the application with necessary information during the development process. Make sure to have these prerequisites in place to streamline the building process of the Uber clone.
How do I set up Google Places Autocomplete in the Uber clone?
To set up Google Places Autocomplete in the Uber clone project, begin by installing the React Native Places Autocomplete library using npm or yarn. Obtain Google Places API keys and activate the Google Places API web service. Create a developer account on Google and access the Google Developers Console. Generate a new application, select it from the dropdown menu, and search for the Google Places API in the library. Enable the Google Places API and create a new API key credential. Import the Google Places Autocomplete component and render it with placeholder text and a query. Verify the installation by running 'npx pod install' for iOS compatibility. Implement the Google Autocomplete component by importing it from the library and adding the API key to the query. Troubleshoot any display issues by adjusting the container's height to ensure proper rendering. Set up a redirect to the results page when both origin and destination places are selected.
What are the steps to implement custom markers in the Uber clone?
To implement custom markers in the Uber clone project, start by installing the React Native Maps library using npm or yarn. For iOS, install pods, enable Google Maps, and update the delegate.m file with an API key. For Android setup, update the build.gradle file with specific versions and add the Google Maps API key in the AndroidManifest.xml file. Test the map view on both iOS and Android devices for compatibility. Render a map view and customize its appearance using flex styles. Implement markers on the map to display cars or other elements by using markers with custom views. Finish the home screen by rendering cards on the map to represent available services or locations. Create a custom marker with a specific image related to longitude and a car image sourced from assets. Utilize the FlatList component to render each car's marker dynamically based on its type and location. Ensure proper functionality by enabling the Directions API for displaying routes between specified origins and destinations on the map.
Related videos
notJust․dev
Build a full stack UBER EATS clone - 3/5 Days Challenge 🔴
notJust․dev
🔴 Netflix Backend in React Native & AWS Amplify (Tutorial for Beginners)
notJust․dev
Build a Realtime Chat App in React Native (tutorial for beginners) 🔴
notJust․dev
Building a Full Stack Workout Tracker with React Native & MongoDB
notJust․dev
Realtime Chat App in React Native and AWS (Backend) 🔴
Summary
00:00
Building Uber Clone in React Native with AWS Amplify
- The project is to build an Uber clone in React Native, with a full-stack application using AWS Amplify.
- The application will feature a home screen with a map displaying the user's location and a message related to COVID.
- A search bar will lead to a destination search page with Google autocomplete integration for address suggestions.
- Users will select from various trip options like UberX, Comfort, and Excel, with displayed prices and a route map.
- Unique features include map directions, Google autocomplete, and a drawer navigator implementation.
- Technologies used include React Native, JavaScript, React functional components, and React hooks.
- Libraries like React Navigation and Vector Icons will be utilized, along with Google APIs for maps and autocomplete.
- Learning points include setting up a React Native project, rendering elements, creating reusable components, and managing navigation.
- Prerequisites include a set React Native environment, a Google developer account with billing enabled, and access to dummy data and assets.
- The project will involve installing Vector Icons, configuring fonts for iOS and Android, and testing the icon display functionality.
24:27
"Developing Interactive Home Screen Components"
- Start by cleaning the project, deleting unnecessary code from app.js, keeping only the safe area.
- Create a folder structure for source files with directories for screens, components, and assets.
- The home screen will feature a map, a message, and an input box for user interaction.
- Initially, display a dummy map before implementing the actual map library.
- Create a home screen component in the screens directory and import it into app.js for rendering.
- Encounter errors in displaying the home screen due to file extension issues and resolve them.
- Develop a separate component for the map in the components directory and import it into the home screen.
- Create a covered message component with styled text and import it into the home screen.
- Implement styling for the covered message component using a separate styles.js file.
- Introduce a bottom component resembling a bottom sheet for user interaction and redirection to a new page.
- Create a home search component with input boxes for previous destinations and home destinations.
- Design the input box with a text field, a clock icon, and a dropdown arrow icon for user interaction.
- Import necessary icons from the assets folder and style the home search component for functionality.
57:52
Styling Input Boxes and Icons in UI
- The text discusses the need to resolve material icons and typos within the focus area.
- Styles for input boxes, input text, and time container are detailed.
- The input box will have a gray background color, margins of 10, and flex direction row for items alignment.
- Input text will have a font size of 20, font weight bold, and a gray color.
- The time container will display items in the same row with a fixed width of 70 pixels and white background color.
- The search container will include rows with icons and destination names.
- Icons will be placed inside an icon container with a white color and a circle design.
- Destination names like "Spin Nightclub" will be styled with a font weight of 600 and a font size of 16.
- The search container will have different background colors for icons to distinguish them.
- The destination search page will feature two input boxes for starting and destination locations, styled with a height of 50, background color of #eee, and padding of 5.
01:28:26
Setting up Google Places Autocomplete in React
- To begin, install the React Native Places Autocomplete library using npm or yarn.
- Obtain Google Places API keys and activate the Google Places API web service.
- Create a developer account on Google and access the Google Developers Console.
- Generate a new application, select it from the dropdown menu, and search for the Google Places API in the library.
- Enable the Google Places API and create a new API key credential.
- Import the Google Places Autocomplete component and render it with placeholder text and a query.
- Verify the installation by running 'npx pod install' for iOS compatibility.
- Implement the Google Autocomplete component by importing it from the library and adding the API key to the query.
- Troubleshoot any display issues by adjusting the container's height to ensure proper rendering.
- Set up a redirect to the results page when both origin and destination places are selected.
02:00:11
Creating "Uber Types" component for ride options.
- The text discusses creating a new component for Uber types, focusing on splitting everything into components.
- The component is named "Uber Types" and includes an index.js file for a functional component.
- Styles are declared to ensure proper styling, with a stylesheet imported as a boilerplate.
- The Uber Types component is imported into the search results and rendered.
- The component is structured to display rows for UberX, Comfort, and UberXL, each with an image, text, and price.
- A custom component named "Uber Type Row" is created to handle the repeated structure of the rows.
- Within the Uber Type Row component, there are three smaller components: image, middle container, and right container.
- Data for the types, including names, prices, and durations, is imported and mapped to render multiple rows with different content.
- A button for confirming the Uber ride is added at the bottom, styled with a black background, white text, and bold font.
02:32:06
Cloning Uber App with React Native Maps
- The text discusses cloning the Uber mobile application in React Native, focusing on implementing the maps feature.
- The process involves installing the React Native Maps library using npm or yarn.
- For iOS, steps include installing pods, enabling Google Maps, and updating the delegate.m file with an API key.
- Android setup involves updating the build.gradle file with specific versions and adding the Google Maps API key in the AndroidManifest.xml file.
- Troubleshooting tips are provided in case of errors during installation.
- Testing the map view on both iOS and Android devices is recommended.
- The text demonstrates how to render a map view and customize its appearance using flex styles.
- Implementing markers on the map to display cars or other elements is crucial for the Uber clone.
- The process involves using markers with custom views to display specific information on the map.
- The final steps include finishing the home screen by rendering cards on the map to represent available services or locations.
03:02:20
Custom markers and dynamic rendering in React.
- The process involves creating a custom marker with a specific image related to longitude and a car image.
- To implement this, expand the map view and import the marker, setting dummy latitude and longitude values.
- Images for the marker can be sourced from assets, like "top/uberx.png," with styling adjustments possible.
- Attempts to style the marker directly may not work, leading to the suggestion of implementing a custom marker with a custom view.
- To display multiple markers, a list of cars with their types and coordinates can be obtained from dummy data.
- Utilizing the FlatList component, each car's marker can be rendered dynamically based on its type and location.
- The process involves mapping through the list of cars and rendering markers for each car individually.
- An error with the Google Maps API key may arise, requiring enabling the Directions API for proper functionality.
- The Directions API allows for displaying routes between specified origins and destinations on the map.
- React Navigation will be implemented in the future to enable seamless navigation between screens in the application.
03:30:16
"Creating Custom API with MongoDB on YouTube"
- MongoDB is a technology used daily by the speaker and may be utilized in the future for creating a custom GraphQL API.
- The speaker plans to demonstrate how to create a custom API using MongoDB on YouTube in the near future.
- All tutorials and live streams by the speaker are available on their YouTube channel, including clones of Instagram, Twitter, Spotify, Airbnb, and Tesla.
- The Tesla clone project is beginner-friendly and allows users to build their first project in React Native in two hours.
- AWS Amplify API offers speed of development and flexibility compared to building a server from scratch, making it ideal for startups and MVPs.
- The speaker recommends using Flexbox for responsive UI design to adjust to different screen sizes.
- Deploying React Native iOS apps on the Apple market requires a Mac OS, but can be done for Android on a Windows OS.

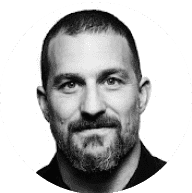
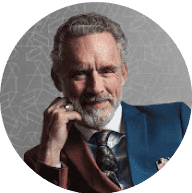
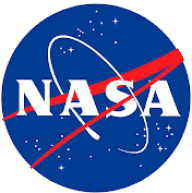
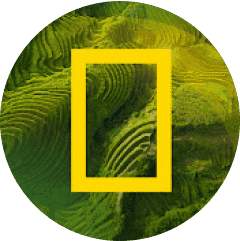