2.8.1 QuickSort Algorithm
Abdul Bari・2 minutes read
Quick sort is an efficient sorting algorithm that organizes elements by selecting a pivot and partitioning the list into smaller segments based on its value, ensuring that elements to the left are smaller and those to the right are larger. The process continues recursively, applying quick sort to the resulting subarrays until the entire list is sorted.
Insights
- Quick sort is an efficient sorting algorithm that organizes elements by allowing them to find their sorted positions independently, starting with a chosen pivot element that partitions the list into smaller segments. This process involves two pointers that swap elements based on their relation to the pivot, ensuring that elements to the left are smaller and those to the right are larger.
- The algorithm employs a recursive approach, applying quick sort to the subarrays created by the pivot's final position. It continues this process until the segments are fully sorted, which occurs when the low index is no longer less than the high index, indicating that the list is either sorted or consists of single elements.
Get key ideas from YouTube videos. It’s free
Recent questions
What is quick sort in simple terms?
Quick sort is a sorting algorithm that organizes elements by selecting a pivot and partitioning the list into smaller segments. It allows each element to find its correct position independently, much like students arranging themselves by height without any external help. The process starts by choosing a pivot element, which is used to divide the list into two parts: those smaller than the pivot and those larger. This method is efficient and works recursively, meaning it applies the same sorting process to the smaller segments until the entire list is sorted.
How does quick sort work step by step?
Quick sort operates in a series of steps that begin with selecting a pivot element from the list. The algorithm then sets up two pointers: one starts at the pivot and the other at the end of the list. It moves the first pointer forward until it finds an element greater than the pivot and moves the second pointer backward until it finds an element smaller than or equal to the pivot. When these pointers meet, the pivot is swapped into its correct position, ensuring that all elements to the left are smaller and those to the right are larger. This partitioning process is repeated recursively for the subarrays created on either side of the pivot until the entire list is sorted.
What are the advantages of quick sort?
Quick sort is favored for its efficiency and speed, particularly with large datasets. One of its main advantages is that it sorts in place, meaning it requires minimal additional memory compared to other sorting algorithms. Additionally, quick sort has an average-case time complexity of O(n log n), making it faster than many other sorting methods. Its recursive nature allows it to handle large lists effectively, and it can be optimized with different pivot selection strategies to improve performance further. Overall, quick sort is a powerful and widely used algorithm in computer science.
When should I use quick sort?
Quick sort is an excellent choice when dealing with large datasets that require efficient sorting. It is particularly useful when memory usage is a concern, as it sorts in place and does not require additional storage for temporary arrays. Quick sort is also preferred when average-case performance is more critical than worst-case performance, as it generally performs well in practice. However, it may not be the best option for small datasets or nearly sorted lists, where simpler algorithms like insertion sort could be more efficient. Overall, quick sort is ideal for scenarios where speed and memory efficiency are paramount.
What is the base case in quick sort?
The base case in quick sort occurs when the algorithm reaches a point where the low index is no longer less than the high index. This indicates that the segments being sorted are either already sorted or contain a single element, which does not require further sorting. At this stage, the recursive calls stop, and the algorithm can begin to return the sorted segments back up the call stack. Recognizing this base case is crucial for the algorithm's efficiency, as it prevents unnecessary processing and ensures that the sorting completes successfully.
Related videos
Ardens
10 FORBIDDEN Sorting Algorithms
TED-Ed
What's the fastest way to alphabetize your bookshelf? - Chand John
Jenny's Lectures CS IT
1.3 Array Operations | Deletion from Array | Explanation with Code | Data Structure
Jenny's Lectures CS IT
1.2 Array Operations - Traversal, Insertion | Explanation with C Program | DSA Course
Yvan Monka
Déterminer une MÉDIANE dans une liste de valeurs - Quatrième
Summary
00:00
Understanding the Quick Sort Algorithm
- Quick sort is a sorting algorithm that allows elements to find their sorted positions independently, similar to students arranging themselves by height without external guidance. The process is initiated by selecting a pivot element, which helps in partitioning the list into smaller segments based on its value.
- The algorithm begins with an initial setup where the list is defined by a low (beginning) and high (end) index, with a placeholder for infinity to represent the end of the list. The pivot is chosen as the first element, and in the example, it is 10.
- The partitioning procedure involves two pointers: one starting from the pivot and the other from infinity. The algorithm increments the first pointer until it finds an element greater than the pivot and decrements the second pointer until it finds an element smaller than or equal to the pivot, allowing for swaps between these elements.
- The process continues until the first pointer exceeds the second pointer, at which point the pivot is placed in its correct sorted position, ensuring that all elements to the left are smaller and all elements to the right are larger than the pivot.
- After the pivot is positioned, quick sort is applied recursively to the subarrays on either side of the pivot. This means the algorithm will sort the left segment (from low to the pivot's position) and the right segment (from the pivot's position + 1 to high).
- The partitioning algorithm is implemented in code, where it takes low and high as parameters, selects the pivot, and uses a loop to continue swapping elements until the pointers meet. The final step involves swapping the pivot with the element at the second pointer's position.
- The quick sort algorithm continues to call itself recursively until the base case is reached, where the low index is no longer less than the high index, indicating that the segments are either sorted or contain a single element.

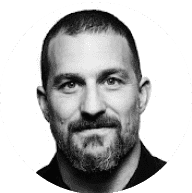
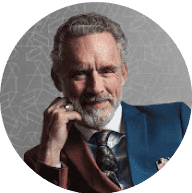
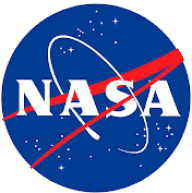
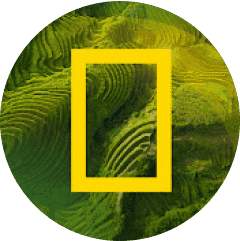