1.2 Array Operations - Traversal, Insertion | Explanation with C Program | DSA Course
Jenny's Lectures CS IT・2 minutes read
The video discusses operations on 1D arrays, such as traversal, insertion, deletion, searching, and sorting, emphasizing the importance of proper initialization and boundary checks in C arrays. Practical examples and detailed explanations guide users through traversing arrays, inserting data, and handling overflow scenarios efficiently, with the best method for insertion being at the end for constant time complexity.
Insights
- Traversal in arrays involves visiting each element once, akin to examining every apple in a row, while insertion requires shifting elements to accommodate new data at the start, end, or a specific position.
- Absence of bounds checking in C arrays necessitates programmers to implement boundary checks, with the process of shifting elements being crucial to maintain array integrity and prevent data loss during insertion operations.
Get key ideas from YouTube videos. It’s free
Recent questions
How are elements inserted in arrays?
Elements can be inserted at the start, end, or specific position in an array.
What is the importance of initializing arrays and variables?
Initializing arrays and variables is crucial for proper data handling.
How does traversal in arrays work?
Traversal involves visiting every element of the array once.
What is the memory allocation for arrays?
Arrays have fixed sizes with memory allocation based on declared size.
How do programmers handle overflow in arrays?
Programmers prevent overflow by checking upper bound limits.
Related videos
Summary
00:00
"1D Array Operations: Traversal, Insertion, Deletion"
- Video focuses on operations performed on 1D arrays in data structures, specifically traversal, insertion, deletion, searching, and sorting.
- Traversal involves visiting every element of the array exactly once, akin to pointing at each apple in a row.
- Insertion in an array can occur at the start, end, or any specific position, with the need to shift elements to accommodate new data.
- Arrays are fixed in size, with memory allocation dependent on the declared size, typically taking four bytes per integer.
- User input determines the size of the array and the elements to be inserted, with the program ensuring no overflow by checking the upper bound limit.
- Traversal and insertion are demonstrated through code examples, emphasizing the importance of initializing arrays and variables.
- The absence of bounds checking in C arrays necessitates programmers to implement boundary checks within their code.
- Inserting data at a specific position involves shifting existing elements to the right to make space for the new value.
- The process of shifting elements is crucial to maintain the integrity of the array and prevent data loss.
- Practical examples and detailed explanations guide users through the steps of traversing arrays, inserting data, and handling potential overflow scenarios.
16:09
Inserting Number at Specific Position in Array
- To insert a number at a specific position in an array, declare variables for the number and position.
- Input the size of the array and its elements, ensuring the size is less than or equal to 50.
- If the size exceeds 50, print an overflow condition; otherwise, proceed with the insertion process.
- Take user inputs for the number to insert and the position, starting the swapping loop from the last element.
- Use a for loop to shift elements to make space for the new number at the desired position.
- Update the array size after inserting the number and print the modified array using a for loop.
- Implement a condition to check the validity of the insertion position, ensuring it falls within the array bounds.
- For inserting at the beginning or end of the array, modify the swapping loop accordingly to shift elements as needed.
- The time complexity for inserting at a specific position in an unsorted array is Theta N, with the best method being to directly insert at the end for constant time complexity.

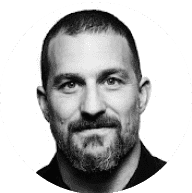
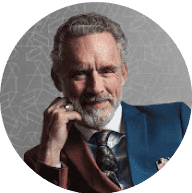
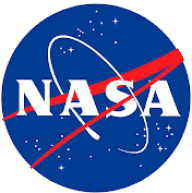
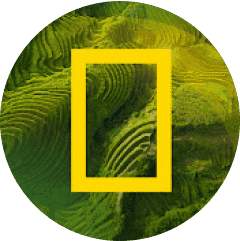