TypeScript in React - COMPLETE Tutorial (Crash Course)
ByteGrad・4 minutes read
TypeScript is essential for React applications, enabling type checking for variables, functions, and components to prevent errors. Various TypeScript features like union types, arrays, type aliases, and helper types streamline development, ensuring type safety and efficient coding practices in React applications.
Insights
- TypeScript is widely used in React applications, allowing for precise typing of variables, functions, and React components, enhancing code readability and preventing errors by specifying prop types and utilizing IntelliSense for props.
- Leveraging features like union types, arrays for props, and type aliases, along with best practices such as employing default values for props and centralizing reusable types in separate files, ensures type safety, flexibility, and efficiency in TypeScript development, ultimately improving code quality and maintainability.
Get key ideas from YouTube videos. It’s free
Recent questions
How does TypeScript enhance React applications?
TypeScript improves React by enabling variable typing, ensuring type safety, and enhancing code readability.
What are the benefits of using type declarations for props in React components?
Type declarations for props prevent errors, enhance code readability, and provide IntelliSense for available props.
How can arrays be utilized as types for props in TypeScript?
Arrays can be used as types for props in TypeScript to allow for passing multiple values efficiently.
Why is it important to specify the type of event handlers in TypeScript and React?
Specifying the type of event handlers in TypeScript and React ensures proper typing and functionality based on the context.
What is the significance of using generics in TypeScript for React components?
Generics in TypeScript establish relationships between props, ensuring consistency in the types of data passed and enhancing type safety.
Related videos
Summary
00:00
"TypeScript in React: Typing Components for Clarity"
- TypeScript has become a standard in React applications.
- Converting a simple button component from JSX to TSX involves enabling TypeScript in the file.
- TypeScript allows for variable typing, inferring types based on assigned values.
- Typing functions in TypeScript involves specifying parameter types to avoid any type.
- React components are essentially functions, with props as parameters.
- Typing React components involves specifying prop types to prevent errors.
- Using a separate type declaration for props in React components can enhance code readability.
- TypeScript provides IntelliSense for available props in React components.
- Union types in TypeScript allow for specifying specific values for props.
- Arrays can be used as types for props in TypeScript, allowing for multiple values to be passed.
11:20
Efficient CSS Management with TypeScript and React
- When specifying pixel values, ensure they are numerical, not in string format, to avoid errors.
- Use tuples to define a specific array structure with a set number of elements and types.
- Utilize arrays of numbers to efficiently manage CSS properties like text color and padding.
- Instead of individually specifying CSS properties, create a single style object for ease.
- Employ React's CSS properties types to streamline the process of passing CSS properties.
- Define specific objects for properties like border radius, with keys and values for each corner.
- Pass functions as props, ensuring correct typing with parameters and return values.
- Utilize the children prop to allow for dynamic content within custom components.
- When passing state setter functions, ensure they accept the correct data type, like numbers.
- Implement default values for props to simplify typing, allowing TypeScript to infer types automatically.
23:17
"TypeScript Tips for React Development"
- Union types are used to combine different types, like a type of color with red, blue, and green.
- Type aliases are preferred over interfaces due to their flexibility and ability to handle various scenarios.
- When creating a button component, attributes like type, autofocus, and others can be specified and accepted as props.
- To allow all attributes of a native element to be passed as props to a custom component, a helper type like `component props` can be used.
- Helper types in React, like `component props`, are namespace-based on React and can be used to manage props efficiently.
- When dealing with event handlers in TypeScript and React, the type of the event can be inferred or explicitly typed based on the context.
- Hooks in TypeScript, like `useState`, can infer types based on initial values, but types can also be explicitly specified if needed.
- Objects in TypeScript require explicit typing, especially when dealing with initial values like `null` and later assigning a specific object type.
- Optional chaining can be used to handle cases where an object may be `null` and prevent potential errors.
- When using hooks like `useRef`, specifying the eventual type of the ref, such as `HTMLButtonElement`, ensures proper typing and functionality.
35:34
Enhancing TypeScript with Advanced Features
- The use of `as const` in TypeScript makes arrays more specific, ensuring they are read-only and contain only specified values.
- The `omit` utility in TypeScript allows for the removal of specific properties from a type, creating a new type without those properties.
- When retrieving data from `localStorage` in React using `useEffect`, type assertion (`as`) can be used to ensure the retrieved data matches a specific type.
- Generics in TypeScript allow for the creation of flexible functions that can work with different types of data, ensuring type safety.
- In React components, generics can be used to establish relationships between different props, ensuring consistency in the types of data passed.
- Centralizing reusable types in a separate TypeScript file, like `types.ts`, allows for easy import and use of those types across multiple components.
- When importing types in TypeScript, specifying `type` in front of the imported type makes it clear that it is a TypeScript type and not a regular JavaScript variable.
- The `unknown` type in TypeScript is useful when the type of a value is not known, providing a safer alternative to using `any` when dealing with uncertain data types in APIs.
47:50
"Data Validation and Automation in Typescript"
- The appropriate type to use is a Known Unknown, which indicates a lack of knowledge about the data, necessitating verification of its shape before use.
- Schemas, like Zod, are crucial for validating data shapes, ensuring compatibility before processing the information.
- The TS reset package from Met Pook automates the conversion of data types from any to unknown, simplifying the process and offering additional benefits.
- Typescript definitions for third-party libraries are often found in the @types repository, providing essential type definitions for frameworks like React and Node.js.

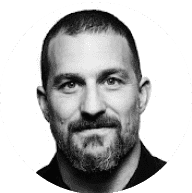
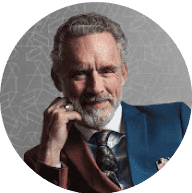
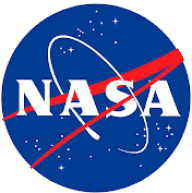
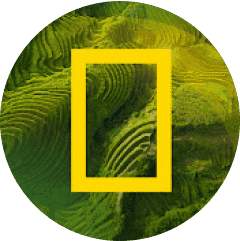