Simple C# Data Access with Dapper and SQL - Minimal API Project Part 1
IAmTimCorey・2 minutes read
.NET 6 now features minimal APIs, with Tim Corey creating a video on setting up SQL databases and configuring Dapper in C# for simple data access. This approach aims to streamline API setup and is applicable beyond minimal APIs, offering a simplified method for C# development.
Insights
- Minimal APIs are now the default in .NET 6, simplifying API setup and focusing on creating a CRUD data layer efficiently.
- Tim Corey's tutorial demonstrates setting up a SQL database and configuring Dapper in C# to streamline data access, applicable beyond minimal APIs, emphasizing simplicity and practicality in C# learning.
Get key ideas from YouTube videos. It’s free
Recent questions
What is the focus of minimal APIs in .NET 6?
Understanding minimal APIs and their applications.
How can I set up a SQL database in C# for data access?
Setting up a SQL database and configuring Dapper.
How can I publish a SQL script in Visual Studio?
Publishing a SQL script in Visual Studio.
What is the role of a SQL data access class in C#?
Communicating with SQL databases through Dapper.
How can I interact with a SQL database indirectly in C#?
Using a separate class for database interaction.
Related videos
IAmTimCorey
SQL Data Tools In C# - Database Creation, Management, and Deployment in Visual Studio
Great Learning
.Net Fundamentals | Introduction to .NET Framework | .NET for Beginners | Great Learning
IAmTimCorey
SQL Stored Procedures - What They Are, Best Practices, Security, and More...
IAmTimCorey
C# Async / Await - Make your app more responsive and faster with asynchronous programming
Web Dev Simplified
Learn SQL In 60 Minutes
Summary
00:00
.NET 6: Minimal APIs with Dapper in C#
- Minimal APIs are now a default in .NET 6.
- The focus is on understanding how minimal APIs work and their real-world applications.
- Two videos will cover the topic, with this one focusing on setting up a SQL database and configuring Dapper in C# for easy data access.
- The goal is to establish a simple CRUD data layer to streamline the API setup in part two.
- The lesson is applicable to various user interfaces in C# beyond minimal APIs.
- Tim Corey aims to simplify C# learning through regular YouTube videos and training courses on timcory.com.
- Source code for the video can be accessed through a link in the description.
- The process begins by creating a new project in Visual Studio for a minimal API demo.
- A SQL Server database project is added to set up a user database with basic specifications.
- Stored procedures are created for CRUD operations, including getting all users, getting one user, deleting a user, inserting a user, and updating a user.
18:25
Efficient SQL Server Post Deployment Script
- Only one post deploy script is allowed, named script.postdeployment.sql.
- Use T-SQL code for SQL Server, with an if statement checking for existing records.
- Use "if not exists" with a select query to determine if records exist.
- Opt for "select 1" over "select top 1" for faster execution.
- Insert sample data into the user table using hard-coded values.
- Multiple rows can be inserted in one statement by separating them with commas.
- The script will not run in production if records already exist in the user table.
- Publish the script by right-clicking and selecting "publish," choosing the local MS SQL Local DB.
- Save the profile for future use and avoid including it in source control.
- Use SQL Server Object Explorer to view the published database and its tables, stored procedures, and data.
36:50
Creating SQL Data Access Class for Communication
- To access data or communicate with SQL Server, right-click on the database access and select "Add New Class" to create a SQL data access class.
- The SQL data access class directly communicates with SQL through Dapper, simplifying the setup process by creating a generic interface.
- Ensure the class is public for external communication and include a constructor that takes in an IConfiguration parameter for dependency injection.
- Utilize the IConfiguration parameter to access data from various sources like appsettings.json, secrets.json, environmental variables, and more.
- Add necessary using statements for System.Data.SqlClient and Dapper to facilitate SQL communication.
- Implement two methods, "load data" and "save data," both as asynchronous tasks to prevent UI lockups during database calls.
- The "load data" method retrieves data from SQL using a stored procedure, parameters, and a connection string.
- The "save data" method executes SQL stored procedures for insert, update, or delete operations, passing parameters and a connection string.
- Extract an interface from the SQL data access class for easy integration with dependency injection.
- Create a new class, "user data," in the data folder to interact with the SQL data access class indirectly, ensuring public access and constructor setup for dependency injection.
56:13
Efficient SQL Data Access Configuration and Methods
- SQL data access configuration involves creating a private read-only configuration and constructor.
- SQL data access interface allows access to load and save data functions.
- User data methods are created for database interaction, focusing on compact code.
- The get users method retrieves all users from the database using a stored procedure.
- Inconsistent accessibility error can occur if return type is less accessible than the method.
- Abstraction around Dapper simplifies the load data method to one line for data retrieval.
- The get user method retrieves a single user based on the provided ID.
- Insert user method inserts a new user into the database using the first name and last name.
- Update user method updates a user's information in the database using the entire user model.

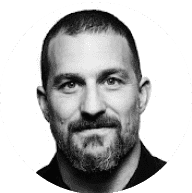
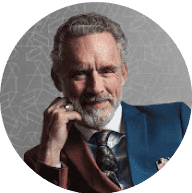
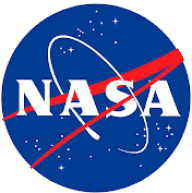
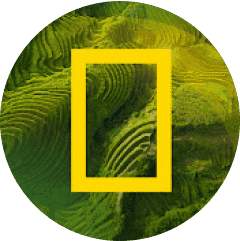