Search - Lecture 0 - CS50's Introduction to Artificial Intelligence with Python 2020
CS50・106 minutes read
Artificial intelligence courses delve into foundational concepts, covering search problems, knowledge representation, uncertainty, optimization, machine learning, and neural networks to enhance computer capabilities effectively. The text transitions to code implementation for DFS, BFS, and informed search algorithms like GBFS and A* in solving mazes, emphasizing their efficiency and optimality in finding solutions in different scenarios.
Insights
- Artificial intelligence encompasses various techniques where computers exhibit intelligent behavior, like recognizing faces or playing games better than humans.
- Knowledge representation is crucial for AI to store information, draw inferences, and make decisions based on existing data.
- Informed search algorithms, like A*, consider heuristic values and steps taken for optimal pathfinding.
- The Minimax algorithm is effective for deterministic games, aiming to maximize or minimize scores in adversarial situations.
Get key ideas from YouTube videos. It’s free
Recent questions
What is artificial intelligence?
Computers exhibiting intelligent behavior like humans.
Related videos
CS50
Knowledge - Lecture 1 - CS50's Introduction to Artificial Intelligence with Python 2020
Stanford Online
Overview Artificial Intelligence Course | Stanford CS221: Learn AI (Autumn 2019)
AI Search
You Don't Understand AI Until You Watch THIS
Simplilearn
Artificial Intelligence Tutorial | AI Tutorial for Beginners | Artificial Intelligence | Simplilearn
Universidad de La Sabana
La inteligenciaartificial y su impacto en la educación
Summary
00:00
"Foundational AI Concepts: Search, Knowledge, Optimization, Machine Learning"
- Artificial intelligence encompasses various techniques where computers exhibit intelligent behavior, like recognizing faces or playing games better than humans.
- The course delves into foundational AI concepts, starting with search problems where an AI seeks solutions, such as finding driving directions or making optimal moves in games like tic-tac-toe.
- Knowledge representation is crucial for AI to store information, draw inferences, and make decisions based on existing data.
- Uncertainty in AI involves dealing with probabilities and uncertain events to enhance decision-making processes.
- Optimization in AI focuses on finding the best solutions to problems by evaluating multiple possible approaches.
- Machine learning enables computers to learn from data and experiences, improving their performance over time, like distinguishing between spam and important emails.
- Neural networks mimic human brain structures, enhancing computer capabilities in performing tasks effectively.
- Language processing in AI involves understanding human languages, presenting challenges and opportunities for AI development.
- Search problems in AI involve finding solutions by navigating through different states and taking actions to reach a goal.
- AI components include agents perceiving environments, states representing configurations, actions as choices in states, and transition models defining state changes after actions.
11:38
Efficiently minimizing path costs in search algorithms.
- In complex problems, there can be multiple possible goals and ways to solve them.
- Computers may aim not just to find a goal but to find it efficiently, with low cost.
- Path cost is a crucial term in defining search problems, representing the expense of each path.
- Search problems often assign numerical costs to paths to minimize expenses or time.
- Search problems involve an initial state, actions, a transition model, a goal test, and a path cost function.
- The goal is to find an optimal solution with the lowest path cost among all possibilities.
- Nodes are data structures used to track states, parents, actions, and path costs in search problems.
- The search algorithm involves exploring options from a state, updating a frontier with available options, and checking for a solution.
- The algorithm repeats the process of removing nodes from the frontier, checking for goals, expanding nodes, and updating the frontier until a solution is found or the frontier is empty.
- Potential issues in the search algorithm can arise when there are bidirectional paths, leading to back-and-forth movements between states.
22:53
Exploring Paths Efficiently: Depth vs Breadth
- The process begins with considering point A and removing it from the frontier, then exploring the options reachable from A.
- After adding choice B to the frontier, the focus shifts to exploring the possibilities from B, including A, C, and D due to a reverse arrow.
- To prevent looping between states, a method of tracking explored states is introduced, ensuring no revisits to already explored nodes.
- The revised approach involves maintaining a frontier with the initial state and an explored set to avoid revisiting nodes.
- Nodes are only added to the frontier if they are not already present in the frontier or explored set, preventing redundant exploration.
- The choice of a stack data structure for the frontier in depth-first search ensures exploration of the deepest nodes first.
- Depth-first search delves deeply into the search tree, backing up when reaching a dead end, exploring one path fully before backtracking.
- In maze-solving scenarios, depth-first search may not always find the optimal solution due to arbitrary path choices.
- Breadth-first search, using a queue data structure, explores the shallowest nodes first, ensuring a more systematic exploration of paths.
- Breadth-first search may lead to finding the optimal solution in maze scenarios by exploring all possible paths systematically.
33:57
"DFS vs. BFS: Maze-solving strategies compared"
- BFS prioritizes shallower nodes first, examining nodes one, two, three, etc., steps away from the initial state.
- DFS, in contrast, follows one path continuously, while BFS explores all possible paths simultaneously, alternating between them.
- BFS ensures to explore shallower nodes earlier, bouncing back and forth between paths to reach the goal optimally.
- The process continues, examining nodes further away until reaching the goal, even if some states explored do not lead to the solution.
- In a larger maze, BFS explores extensively, potentially requiring exploration of numerous states to find the optimal path.
- The text transitions to code implementation for DFS and BFS in solving mazes, utilizing classes like 'node' and 'stack frontier'.
- The 'stack frontier' class manages frontier data, adding, checking, and removing nodes as a stack structure.
- An alternative 'Q frontier' class is introduced, inheriting from 'stack frontier' but removing nodes differently.
- The 'maze' class handles maze-solving processes, parsing maze text files and implementing the solve function.
- The solve function utilizes a stack frontier to explore states, backtrack from the goal, and find the optimal path, as demonstrated in maze1.txt and maze2.txt examples.
45:48
Efficient Exploration with Informed Search Algorithms
- Modifying the program by adding an argument called "show explored" and setting it to true highlights all states explored from initial to goal in red.
- Depth-First Search (DFS) explores paths extensively, sometimes leading to unnecessary states being explored before finding the optimal solution.
- Breadth-First Search (BFS) explores closer states first, resulting in fewer states explored compared to DFS.
- BFS and DFS may find the same solution in some cases, but not always, as shown in maze3.txt.
- DFS may not always find the optimal solution, as it exhausts one path before trying another.
- Informed search algorithms, like Greedy Best-First Search (GBFS), use problem-specific knowledge to find solutions more efficiently.
- GBFS expands nodes closest to the goal based on a heuristic function, such as the Manhattan distance in maze-solving.
- The heuristic function estimates the distance to the goal, helping the algorithm choose the most promising nodes to explore.
- By using the Manhattan distance heuristic, GBFS can prioritize nodes closer to the goal, leading to more efficient exploration.
- Informed search algorithms, like GBFS, offer a more intelligent approach to problem-solving by considering specific knowledge about the problem domain.
57:48
Optimal Pathfinding with A* Search Algorithm
- The heuristic function assigns values based on Manhattan distance from cells to the goal.
- The Manhattan distance is calculated by the number of squares moved horizontally and vertically to reach the goal.
- The heuristic function estimates proximity to the goal but may not always reflect the actual number of steps required.
- Greedy best-first search algorithm prioritizes nodes with the smallest heuristic values.
- Decision points in the algorithm involve choosing nodes based on their estimated distance from the goal.
- Greedy best-first search may not always find the optimal path due to its local decision-making approach.
- A* search algorithm considers both the heuristic value and the steps taken to reach a node for decision-making.
- A* search aims to find the optimal path by summing the steps taken and the heuristic value for each node.
- A* search algorithm can lead to optimal solutions by considering both the heuristic estimate and the steps taken.
- A* search is optimal under certain conditions, requiring an admissible heuristic function.
01:09:42
Optimal Heuristics in Search Algorithms
- A heuristic is admissible if it never overestimates the true cost in search algorithms.
- The heuristic value should either be exact or underestimate the distance to the goal.
- Consistency in the heuristic is crucial, ensuring that for every node and successor, the heuristic value of the node is less than or equal to the successor's heuristic value plus the cost.
- A* search algorithm requires a consistent heuristic to find an optimal solution.
- The choice of heuristic is a significant challenge in solving search problems.
- A* search algorithm can be memory-intensive, leading to alternative approaches with lower memory usage.
- Adversarial situations in games like tic-tac-toe involve intelligent decision-making against an opponent with opposing objectives.
- The Minimax algorithm is effective for deterministic games with two players, aiming to maximize or minimize scores.
- Translating game concepts into numerical values is essential for AI understanding.
- Game encoding for AI includes defining initial state, player function, actions, transition model, terminal state, and utility function.
01:21:13
"Minimax Algorithm: Maximizing and Minimizing Strategies"
- A state S is defined where a game not yet over, when passed into the terminal function, outputs false, indicating the game is ongoing.
- Conversely, a game that is over, such as when X achieves three in a row along a diagonal, when passed into the terminal function, outputs true, signifying the game's conclusion.
- The AI is informed of the game's rules, valid moves, and conditions for game completion.
- To determine the value of each state, a utility function is defined, assigning a value of 1 for X winning and -1 for O winning.
- The Minimax algorithm is introduced, where X aims to maximize the score while O aims to minimize it.
- In a game mid-progress, O's turn is identified using the player function, and the value of the board is calculated based on possible moves.
- Minimax recursively evaluates possible actions and their resulting states, considering both maximizing and minimizing perspectives.
- The algorithm involves predicting opponents' moves and selecting actions that lead to the most favorable outcomes.
- A generic Minimax tree is illustrated, showcasing maximizing and minimizing states based on players' objectives.
- Pseudocode outlines the Minimax algorithm, detailing how the max player selects actions for maximum value and the min player for minimum value, considering opponents' responses.
01:32:32
Enhancing Minimax Efficiency with Alpha-Beta Pruning
- The Minimax algorithm involves implementing a maxValue function to maximize the value of a state.
- If the game is over, the utility function determines the value of the board.
- In cases where the game continues, recursive reasoning is needed to predict the opponent's next move.
- The value of the state is tracked in a variable called v, initially set to negative infinity.
- A loop iterates over possible actions, comparing them to v to maximize the score.
- The minValue function calculates the minimum value of a state, following similar logic but in reverse.
- Alpha-beta pruning is an optimization technique to minimize unnecessary calculations in the Minimax algorithm.
- By pruning branches with values lower than the current best option, unnecessary computations are avoided.
- This optimization allows for more efficient decision-making by discarding paths that cannot improve the current best option.
- Alpha-beta pruning enhances the Minimax algorithm's efficiency, especially in complex games with multiple possible moves.
01:43:34
Optimizing Search Processes in Adversarial Games
- Alpha-beta pruning is a technique to optimize search processes by removing unnecessary nodes in a search tree, making searches more efficient.
- Tic-tac-toe has approximately 255,000 possible games, while chess can have over 10 to the 29,000 possible games, posing a challenge for the Minimax algorithm due to the vast number of states to consider.
- Depth-limited Minimax is a more practical approach than the traditional Minimax, limiting the search depth to a certain number of moves to avoid computational intractability.
- An evaluation function is crucial in depth-limited Minimax to assign a score to game states, estimating the expected utility of a game from a given state.
- Various variants of Minimax exist, incorporating additional features like evaluation functions to enhance performance in complex and computationally challenging scenarios, aiding AI in making better decisions in adversarial search problems.

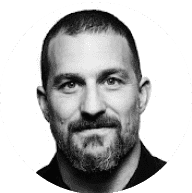
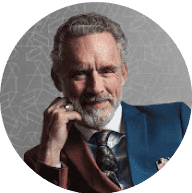
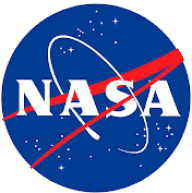
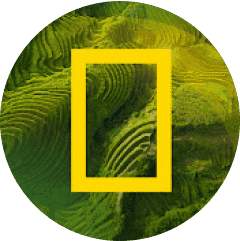