PHP Full Course for non-haters π
Bro Codeγ»11 minutes read
PHP is a widely-used scripting language for web development, despite claims of its demise, with a strong presence on websites. It encompasses basic concepts, such as variables, operators, loops, arrays, forms, conditions, functions, user input validation, and database connectivity, making it crucial for dynamic web applications.
Insights
- PHP remains prevalent, powering 70% of websites due to its speed, simplicity, and flexibility.
- PHP's evolution from Personal Home Page to PHP Hypertext Preprocessor highlights its organic growth.
- A solid foundation in HTML and MySQL is essential to start working with PHP effectively.
- PHP files offer versatility by allowing the inclusion of HTML, CSS, JavaScript, and PHP code within a single file.
- The security benefits of using the post method over get in PHP forms are crucial for preventing URL manipulation.
Get key ideas from YouTube videos. Itβs free
Recent questions
What is PHP used for?
PHP is a server-side scripting language for dynamic web pages.
Related videos
Summary
00:00
PHP: Dynamic, Fast, and Versatile Web Development
- PHP is a server-side scripting language used for building dynamic web pages, running on servers, not user browsers.
- Despite claims of PHP being dead, it is still used on 70% of known websites, valued for its speed, simplicity, and flexibility.
- Originally an acronym for Personal Home Page, PHP evolved into PHP Hypertext Preprocessor since its release in 1995.
- PHP was not initially intended as a new programming language but grew organically to its current status.
- PHP's mascot is an elephant named Elephant Ella, reflecting its organic growth.
- PHP basics involve a browser sending a request to a server, PHP processing it, and the server sending HTML back to the browser.
- PHP commonly works with relational databases like MySQL, Postgres, and Oracle, often written alongside HTML.
- To start working with PHP, a solid foundation in HTML is necessary, along with knowledge of MySQL.
- Recommended tools for PHP development include XAMPP for a web server solution stack and Visual Studio Code as a text editor.
- PHP files can contain HTML, CSS, JavaScript, and PHP code, allowing for versatile web development within a single file.
21:04
PHP Basics: Floats, Booleans, Variables, Operators
- A float in PHP represents a price with a decimal portion for cents, like a pizza priced at 4.99.
- To display a dollar sign before a float value, use an escape sequence by preceding the dollar sign with a backslash.
- Another example of a floating-point number is a tax rate, like a sales tax rate of 5.1 percent.
- Booleans in PHP are either true or false, representing binary states like employed or not employed, online or offline, for sale or not for sale.
- When displaying Boolean values as output, true is represented by 1 and false by 0, usually used internally in programs.
- Variables in PHP are containers for data, including strings, integers, floats, and booleans.
- Arithmetic operators in PHP include addition, subtraction, multiplication, division, exponents, and modulus for remainder.
- Increment and decrement operators like ++ and -- can be used to increase or decrease variables by one or a specified value.
- Operator precedence in PHP dictates the order of solving complex arithmetic expressions, starting with parentheses, then exponents, multiplication, division, and addition/subtraction.
- Get and post variables in PHP are used to collect data from HTML forms, with get appending data to the URL and post sending data securely in the body of the HTTP request.
41:54
Creating PHP Forms for User Input and Calculations
- To create a form, use a pair of form tags and set the action attribute to a file location, sending data to index.php using the post method.
- Include a text box for quantity within the form, labeling it as such, and adding a line break.
- In the PHP script, define variables for a food item (like pizza) and its price (e.g., $5.99), calculating the total based on the quantity input by the user.
- Utilize local variables to store values from the HTML form for easier manipulation.
- Display an order total message including the quantity, item name, and total price.
- Highlight the security benefits of using the post method over get, preventing URL manipulation.
- Explain the process of creating a form in HTML to accept user input for mathematical functions in PHP.
- Demonstrate the use of math-related functions like abs, round, floor, ceil, power, sqrt, max, min, pi, and rand with practical examples.
- Present an exercise involving user input for radius calculation of circumference, area, and volume of a circle or sphere using PHP.
- Illustrate the concept of if statements in PHP, showing how to check conditions like age being above 18 and providing different messages based on the outcome.
01:03:37
Programming Conditions and Logical Operators Explained
- Conditions in programming determine the execution of code based on true or false statements.
- The order of if and else if statements impacts code execution.
- Boolean variables can be used in conditions to determine actions.
- A shorthand for comparing Boolean variables is using the variable name directly.
- An example program calculates pay based on hours worked and hourly rate.
- Overtime pay is calculated if hours exceed 40, following specific formulas.
- Logical operators like and, or, and not can combine conditions for more complex checks.
- An example with temperature ranges demonstrates the use of logical operators.
- Switch statements are more efficient than multiple else if statements for handling multiple conditions.
- Switch statements allow for cases to be defined for different scenarios, with a default case for unmatched conditions.
01:25:01
"Switch and Loop Statements for Efficiency"
- Break statements are crucial in switch statements to exit the switch after a match; without them, subsequent code will execute.
- Including break statements in switch cases is essential to avoid executing all statements following a match.
- An example demonstrates using a switch statement based on the current date to display different messages for each day of the week.
- The switch statement allows for custom messages based on the day of the week, with specific cases for each day.
- A default case can be added to handle scenarios where no matching cases are found in the switch statement.
- Switch statements are recommended for efficiency when checking values or variables multiple times.
- For loops are used to repeat code a specific number of times, with the ability to set a counter, condition, and increment or decrement statements.
- The for loop example showcases counting up to a specified number and customizing the increment value.
- An exercise demonstrates creating an HTML form in VS Code using a for loop to count up to a user-input number.
- While loops are introduced as a way to repeat code indefinitely while a condition remains true, with the need for a manual escape mechanism.
01:46:01
Array Manipulation Techniques
- To display all elements in an array, use a for each Loop.
- To change an element in an array, type the array name followed by an index number.
- Use the push function to add a new element to the end of an array.
- The pop function removes the last element in an array.
- The shift function removes the first element in an array.
- Reverse an array using the array_reverse function.
- Count the elements in an array using the count function.
- Associative arrays consist of key-value pairs.
- Use isset to check if a variable is declared and not null.
- Use empty to check if a variable is not declared, false, null, or an empty string.
02:09:24
PHP Code: User Input Validation and Functions
- To display a welcome message, the code checks if the username is missing; if so, it prompts the user to input a username.
- Upon pressing login without a username, the system detects the missing username and prompts the user to input it.
- An "else if" statement is added to check for a missing password; if the password is empty, the system prompts the user to input a password.
- To output a hello message, both a username and a password are required; the system prompts the user to input both.
- Enclosing a code section within a comment block is demonstrated, and the process is explained.
- The code utilizes the post super global variable, containing key-value pairs, to check if the login button is set and if the username and password fields are empty.
- A demonstration of working with radio buttons in PHP is provided, including creating radio buttons, assigning values, and using the post variable to access the selected option.
- A switch statement is used to compare the selected radio button value and display a corresponding message.
- The process of working with checkboxes in PHP is explained, including creating checkboxes, checking if they are set or empty, and placing them in an array.
- Functions in PHP are introduced, with an example of creating a "Happy Birthday" function that can be called multiple times with different arguments.
02:30:27
Function Parameters and Return Values Explained
- To pass a parameter within a function, it exists only within that function's scope; escaping the function removes the variable.
- Replace 'U' with a placeholder, then add the parameter 'first name' for a birthday message.
- Demonstrates invoking a function with different arguments like 'SpongeBob', 'Patrick', and 'Squidward'.
- Passing more than one argument to a function, like an age, separated by commas.
- Illustrates assigning ages to 'SpongeBob' (30), 'Patrick' (35), and 'Squidward' (45) within a function.
- Explains the necessity of matching parameters when invoking a function with arguments.
- Introduces the concept of returning a value from a function, typically seen at the function's end.
- Creating a function to determine if a number is even or odd using the modulus operator.
- Developing a function to find the hypotenuse of a right triangle using the square root function.
- Discusses the importance of declaring data types for parameters to ensure accurate inputs.
02:51:14
"Validate input, sanitize user input in PHP"
- To validate input, first check if it consists solely of numbers.
- Next, validate an email's correct format by copying and pasting two lines of code.
- Assign the user's email input to a variable named "email."
- Validate the email input using a filter; if empty, the email is invalid.
- Demonstrate the process by inputting an email with illegal characters.
- Emphasize the importance of sanitizing and filtering user input to prevent malicious scripts.
- Illustrate how to sanitize and validate user input in PHP.
- Explain the "include" function in PHP, which copies and includes file content.
- Detail the benefits of using the "include" function for reusable website sections.
- Provide a step-by-step guide on creating header and footer files for a website using the "include" function.
03:13:14
Creating Secure Login System in PHP
- The text discusses creating a login system in PHP, starting with handling empty fields and displaying appropriate messages.
- It introduces the header function to redirect users to the home page after login.
- The process of logging out is detailed, including using the session destroy function.
- The server super Global variable is explained, containing essential information about the web page environment.
- PHP self and request method keys within the server super Global variable are highlighted for file path and form submission detection.
- Hashing in PHP is explained as a method to transform sensitive data like passwords into secure formats.
- The password hash function and password verify function are demonstrated for hashing and verifying passwords.
- Connecting to a MySQL database using the mysqli extension is discussed, including creating a database in phpMyAdmin.
- A separate PHP file for managing database connections is created, with exception handling for connection errors.
- The text emphasizes practical steps for creating a login system, hashing passwords, and connecting to a MySQL database in PHP.
03:33:32
PHP Database Connection and Data Manipulation Tutorial
- The process involves generating HTML after a PHP script in the body, including a "hello" message.
- Database connection is handled in a separate PHP file, which is included in the index page using the include function.
- A message indicating successful database connection is displayed as "you are connected."
- An additional line break is added after the "could not connect" message for better formatting.
- Although unnecessary, informing the user of the database connection status is done for testing purposes.
- In case of connection issues, a message stating "could not connect" is displayed.
- Instructions on creating a table using phpMyAdmin are detailed, including setting columns for user IDs, usernames, passwords, and registration dates.
- The process of inserting rows manually into the table using phpMyAdmin is explained, including setting values for usernames, passwords, and registration dates.
- The method of inserting data into a MySQL table using PHP is outlined, including writing an SQL query, submitting it, and handling exceptions.
- Retrieving data from a MySQL database using PHP is demonstrated, involving writing an SQL query, executing it, and displaying the results, with handling for cases of no results found.
03:54:44
Secure User Registration with Hashing and Exception Handling
- To securely register users in a database, first, hash the password using a hash function after entering the username and password. Then, create an SQL query to insert the username and hashed password into the database table, ensuring unique user IDs to avoid duplicate entries.
- In case of a duplicate entry error, implement exception handling by enclosing the code that may cause an exception in a try block, catching the MySQL exception, and informing the user that the username is already taken to manage registration conflicts effectively.

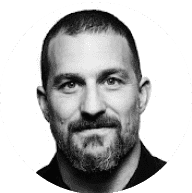
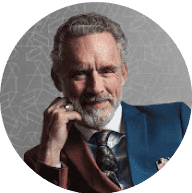
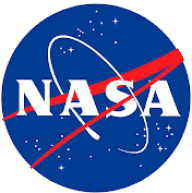
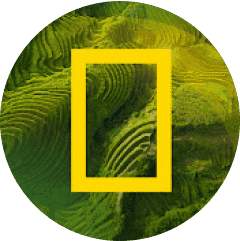