Data Structure 02 | Data Structure (Part 02) | CS & IT | DA | GATE 2025 Crash Course
GATE Wallah - EE, EC, CS & IN・2 minutes read
The session focuses on addressing learning backlogs in data structures, specifically covering tree traversal methods and their applications in programming. Students are encouraged to engage actively with the material, understand the key concepts of binary search trees, and participate in upcoming lectures and assignments to reinforce their learning.
Insights
- The session is designed to help students overcome learning backlogs, particularly focusing on key concepts in data structures like tree traversal, with a follow-up lecture scheduled for Monday after a break.
- The instructor, through practical examples, explains the "floor value" concept, which rounds down numbers, and emphasizes its significance in programming, aiding students in grasping foundational mathematical principles necessary for coding.
- Tree traversal methods, including in-order, pre-order, and post-order, are introduced, with a mnemonic technique suggested to help students visualize and remember the traversal sequences, enhancing their understanding of tree structures in data processing.
- Active engagement is encouraged, as the instructor contrasts passive learning methods with the benefits of thorough reading and comprehension, stressing the importance of collaborative problem-solving and practical exercises to solidify understanding of binary tree concepts.
- Participants are assigned homework related to binary search trees, with a crash course planned for the next month to further address backlogs, ensuring that students remain engaged and prepared for upcoming discussions on complex topics.
Get key ideas from YouTube videos. It’s free
Recent questions
What is a binary search tree?
A binary search tree (BST) is a data structure that organizes data in a hierarchical manner, where each node has at most two children referred to as the left and right child. In a BST, the left child contains values less than its parent node, while the right child contains values greater than its parent node. This property allows for efficient searching, insertion, and deletion operations, as the tree can be traversed in a systematic way. The structure of a BST ensures that operations can be performed in logarithmic time on average, making it a popular choice for implementing dynamic sets and lookup tables.
How do you calculate the height of a tree?
The height of a binary tree is defined as the length of the longest path from the root node to a leaf node. To calculate the height, one can use a recursive approach where the height of each subtree is determined, and the maximum height is taken. For a binary tree with 'n' nodes, the maximum height can be calculated using the formula h = n - 1, which indicates that the height is one less than the total number of nodes in the case of a skewed tree. Conversely, the minimum height can be approximated using the floor value of log2(n), which provides a more balanced view of the tree's structure.
What is tree traversal?
Tree traversal refers to the process of visiting all the nodes in a tree data structure in a specific order. There are several methods of traversal, including in-order, pre-order, and post-order. In-order traversal visits nodes in the order of left child, root, and then right child, which results in sorted output for binary search trees. Pre-order traversal visits the root first, followed by the left and right children, while post-order traversal visits the left and right children before the root. Understanding these traversal methods is crucial for performing operations such as searching, inserting, and deleting nodes in a tree.
What is the floor value in programming?
The floor value in programming is defined as the greatest integer that is less than or equal to a given number. It is commonly used in mathematical computations and algorithms where rounding down is necessary. For example, the floor value of 4.9 is 4, as it is the largest integer not exceeding 4.9. In programming languages, functions like `floor()` are often provided to compute this value easily. The concept of floor value is particularly relevant in scenarios involving data structures, such as determining the height of a binary tree or calculating indices in arrays.
How do you reconstruct a binary tree?
Reconstructing a binary tree typically involves using two types of traversal sequences: post-order and in-order. The last element of the post-order sequence indicates the root of the tree, while the in-order sequence helps identify the left and right subtrees. The process begins by identifying the root from the post-order sequence, then dividing the in-order sequence into left and right parts based on the root's position. This recursive approach continues until the entire tree structure is established, ensuring that each node is placed correctly according to the properties of binary trees. Practicing this reconstruction with various sequences solidifies understanding of tree structures.
Related videos
HK_OFFICIAL_
FDS UNIT-1 Complete ONE SHOT 🔥| Introduction to Algorithms & Data Structures | SPPU Second Year |
Harvard University
Advanced Algorithms (COMPSCI 224), Lecture 1
Xylem Plus One
Plus One Maths - Sets - One Shot Revision | Xylem Plus One | Xylem Plus One
JEE Nexus by Unacademy
Binomial Theorem Class 11 | One Shot | JEE Main & Advanced | Arvind Kalia Sir
Xylem class 9
Class 9 Mathematics - Mega Marathon | Xylem Class 9
Summary
00:00
Mastering Tree Traversal and Learning Strategies
- The session aims to address and resolve backlogs in learning, with a focus on important concepts, particularly in data structures, as part of a "Backlog Killer" series. The second lecture will take place after a break, resuming on Monday.
- The instructor plans to cover the concept of "floor value," which is defined as the greatest integer less than or equal to a given number, using examples like rounding down 4.9 to 4 and explaining its relevance in programming.
- Tree traversal is introduced as the process of visiting every node in a tree structure, with an emphasis on understanding its importance in data processing and computation.
- The instructor provides a practical example of calculating the size of a folder containing subfolders, illustrating that the total size is determined by summing the sizes of the subfolders and the contents of the main folder.
- Three types of tree traversal are outlined: in-order, pre-order, and post-order, with a brief explanation of each: in-order (left-root-right), pre-order (root-left-right), and post-order (left-right-root).
- A shortcut technique for remembering traversal orders is introduced, using a visual method of tracing an outer line around a tree structure to determine the order of node visits.
- The instructor encourages students to visualize the traversal process, suggesting they draw points for left, right, and root nodes to aid in understanding the traversal orders.
- The session emphasizes the importance of structured study, recommending that students read books in a systematic order, starting from the title and abstract, progressing through chapters and sections.
- The instructor highlights the need for students to engage with their studies actively, contrasting the current trend of passive consumption of content (like watching videos) with the benefits of thorough reading and comprehension.
- Practical programming applications of tree traversal are discussed, with the instructor providing tips for efficiently implementing traversal algorithms without extensive programming knowledge, focusing on understanding the concepts rather than just coding.
18:48
Reconstructing Binary Trees from Traversal Sequences
- Post-order traversal is identified by the sequence: left, right, root, where the last element in the sequence is the root of the tree.
- To reconstruct a binary tree, both post-order and in-order traversals are required; the last element of the post-order sequence indicates the root, while the in-order sequence helps identify the left and right subtrees.
- For example, given post-order "CBF" and in-order "DBEAC", the root is "A", with "DBE" on the left and "C" on the right.
- The process involves recursively identifying the root from the post-order, then dividing the in-order sequence into left and right parts based on the root's position.
- The left subtree is constructed from the elements before the root in the in-order sequence, while the right subtree consists of elements after the root.
- For instance, if the post-order is "DGEAB" and in-order is "DBGEAC", the root is "A", with "DBG" on the left and "E" on the right.
- The reconstruction continues recursively, identifying roots and dividing sequences until the entire tree structure is established.
- It is crucial to remember that the last element in the post-order sequence is always the root, and the in-order sequence will always have the root in the middle.
- The traversal methods can be practiced by writing down the sequences and identifying roots and subtrees, ensuring a clear understanding of the tree structure.
- To solidify the concept, practice reconstructing trees from various post-order and in-order sequences, confirming the accuracy of the resulting tree structure.
40:31
Binary Tree Concepts and Collaborative Learning
- The discussion revolves around an order placed by Dev Goswami, with specific sequences mentioned for post-order traversal: 36, 165, 18, 45, 14, 20, 64, and 13, indicating a binary tree structure.
- The participants are asked to determine the number of leaf nodes in the binary tree based on the provided post-order and in-order sequences, emphasizing the need for an integer-type answer.
- A formula is introduced for calculating the maximum height of a binary tree, where the maximum height (h) is given by the formula h = n - 1, resulting in a maximum height of 14 for 15 nodes.
- For the minimum height, participants are instructed to use the floor value of log2(n), where n is the number of nodes, leading to a minimum height of approximately 3.9 for 15 nodes, which rounds down to 3.
- The conversation includes a poll where participants submit their answers regarding the number of leaf nodes, with a total of 70+ children participating and several providing correct answers.
- The process of constructing a binary tree from post-order and in-order sequences is discussed, highlighting the importance of identifying the root and dividing the sequences accordingly.
- The concept of post-fix notation is introduced, explaining how to create an expression tree from a post-fix expression, with examples provided for clarity.
- The participants are encouraged to engage with the material actively, with reminders to write their answers in the chat and to participate in polls for feedback.
- The discussion also touches on the challenges faced by some participants in understanding post-fix expressions, with offers of additional explanations and support.
- Overall, the session emphasizes collaborative learning, problem-solving, and the application of binary tree concepts in practical scenarios, with a focus on clarity and understanding among participants.
01:01:53
Understanding Binary Search Trees and Operations
- The discussion begins with a reference to a completed process involving an "expo antenna," indicating a sequence of operations that have been finalized from 6 to 6, followed by multiplication where the number six is transformed into seven and eight through operations on the left and right.
- The introduction of addition is noted, where the number six is assigned to the left and a new number is assigned to the right, culminating in the introduction of subtraction, which is acknowledged as part of the ongoing process.
- A new order is suggested, emphasizing the importance of understanding the root concept, where the first element is identified as the root, and subsequent elements are placed based on their value relative to the root.
- The explanation includes a method for determining the placement of numbers in a binary search tree, where numbers less than the root go to the left and those greater go to the right, ensuring that this rule applies to all subtrees.
- An example is provided with the numbers 5, 2, 1, 3, and 7, illustrating how to construct a binary search tree by comparing each number to the root and placing it accordingly.
- The concept of unique keys in a database management system (DBMS) is introduced, stressing that each key must be distinct to maintain the integrity of the binary search tree structure.
- A practical exercise is presented, where participants are asked to insert a series of numbers (e.g., 56, 26, 21, 39, 57, 60, 71, 72, 73, 12, 19, 81) into a binary search tree, reinforcing the understanding of left and right subtree dynamics.
- The importance of recognizing the number of nodes in both left and right subtrees is highlighted, with a formula provided (x + y) to calculate the total number of nodes based on their placement.
- The session includes a discussion about the potential confusion that may arise from the stacking of elements and the need for clarity in teaching new concepts, particularly in binary search trees.
- Finally, a question is posed regarding the construction of a binary search tree with specific values, prompting participants to engage with the material actively and apply their understanding of the concepts discussed.
01:22:46
Understanding Binary Trees and Traversal Methods
- The discussion revolves around a binary tree structure, where 11 elements have been removed from a total of 100, leaving 89 elements remaining in the tree. The calculation is presented as 100 - 11 = 89, confirming the number of elements left.
- The speaker emphasizes the importance of understanding the values of x and y, where x equals 11 (the number of elements removed) and y equals 89 (the remaining elements), leading to the conclusion that the total remains 100 when combined.
- Acknowledgment is made of the complexity of the problem, but the speaker reassures that the answer is indeed 100, despite initial confusion, and encourages a clear understanding of the tree structure.
- The speaker introduces the concept of multiple-choice questions related to the insertion sequence in a binary search tree (BST), indicating that there can be more than one valid answer based on the order of insertion.
- The process of inserting elements into the tree is explained, with specific examples given for how elements are placed based on their values, such as inserting 5, 4, 9, 12, and 20, and how they relate to each other in the tree structure.
- The speaker discusses the importance of understanding traversal methods in binary search trees, specifically pre-order and post-order traversals, and instructs students to write down these traversals for further discussion.
- A series of example values for pre-order and post-order traversals are provided, including sequences like 12, 18, 24, 27, 28, and 200, with the speaker asking for confirmation of the correctness of these sequences.
- The speaker highlights the significance of maintaining distinct values in the tree to ensure an increasing order during traversal, explaining that distinct values lead to a clear and organized structure.
- The concept of left and right subtree relationships is reiterated, where the left subtree contains lesser values and the right subtree contains greater values, emphasizing the importance of this structure in maintaining the properties of a binary search tree.
- Finally, the speaker prepares to pose a challenging question to the students, indicating that the upcoming question will test their understanding of the discussed concepts and encourage them to apply their knowledge practically.
01:42:08
Understanding Valid Preorder Traversal in Trees
- The discussion revolves around the concept of valid preorder traversal in binary trees, emphasizing that the first element must be the root, followed by left children (lesser values) and right children (greater values), ensuring a clear sequence without mix-ups.
- A specific example is given with the number 786, which is questioned for its adherence to the traversal rules; it is noted that if 786 is positioned incorrectly (as a left child when it should be greater), it does not follow the rules of valid traversal.
- The instructor mentions that there will be no lecture the following day, with classes resuming on Monday, and outlines a schedule where subjects will be covered daily for a month, emphasizing that there will be no multiple answers to the questions posed.
- Students are assigned homework consisting of questions from 2024, specifically related to binary search trees (BST), and are instructed not to share answers with classmates who may not have seen the questions yet.
- A crash course is announced for the following month, aimed at clearing backlogs in subjects, with a focus on important topics such as binary trees and heaps, and students are encouraged to maintain their energy and participation levels during classes.
- The session concludes with a reminder that students will receive a PDF of the materials discussed, and they are encouraged to attend the next class on Monday to continue with the backlog topics.

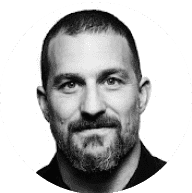
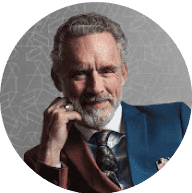
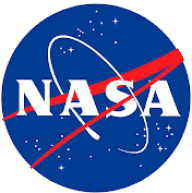
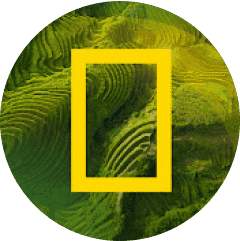