Como sair do ZERO em JAVA em 1h - com @kipperdev
Rocketseat・2 minutes read
Fernanda Kiper introduces Java language in a video tutorial covering basics like installing, configuring, syntax learning, and object-oriented programming concepts, emphasizing Java's platform independence, strong typing, object-oriented nature, and memory management within Java Virtual Machine, with detailed instructions on installing Java, verifying the installation, and utilizing IntelliJ IDE for Java development, along with explanations on variable declarations, data types, conditional statements, arrays, loops, casting, classes, inheritance, polymorphism, and compiling and executing programs efficiently.
Insights
- Java is a strongly typed, object-oriented, and platform-independent language, focusing on data organization in objects with values and functions. The Java Virtual Machine (JVM) interprets and executes compiled Java code, managing memory, garbage collection, and program execution while ensuring platform independence.
- Java programming involves optimizing memory usage by selecting appropriate data types for variables, understanding conditional statements, declaring arrays, utilizing ArrayLists for dynamic arrays, working with loops, casting data types, modeling objects with classes and constructors, controlling method visibility with access modifiers, implementing inheritance, and demonstrating polymorphism through method overrides in different classes.
Get key ideas from YouTube videos. It’s free
Recent questions
What is Java's platform independence?
Java is platform-independent, allowing programs to run on various machines without compatibility issues. This means that Java code can be compiled into bytecode that can run on any device with a Java Virtual Machine (JVM), ensuring consistency across different platforms.
How does Java manage memory?
The Java Virtual Machine (JVM) manages memory, garbage collection, and execution of Java programs. It interprets and executes compiled Java code, ensuring platform independence and efficient memory management by handling memory allocation and deallocation through garbage collection.
What is the purpose of declaring variables in Java?
Declaring variables in Java is essential for specifying the data type and allocating memory space for storing values. By declaring variables, programmers ensure type safety, prevent errors, and optimize memory usage by choosing appropriate data types based on the value being stored.
How does Java support conditional statements?
Java supports conditional statements like if, else, and else if, enabling programmers to execute specific actions based on defined conditions. These statements follow a syntax similar to other programming languages, allowing for the implementation of decision-making logic in Java programs.
What is the significance of polymorphism in Java?
Polymorphism in Java allows objects of different classes to respond to the same message in different ways. It enables flexibility and extensibility in object-oriented programming by facilitating the implementation of methods that can be overridden in subclasses to provide unique behaviors while maintaining a consistent interface.
Related videos
freeCodeCamp.org
Java Programming for Beginners – Full Course
Apna College
Functions & Methods | Java Complete Placement Course | Lecture 7
Apna College
Java OOPs in One Shot | Object Oriented Programming | Java Language | Placement Course
Sir Tarun Rupani
Library Classes in Java | Computer Application ICSE Class 10 | @sirtarunrupani
College Wallah
Java in One Shot | Revision - 1 | Output, Input, Variables, Operators | Java Course
Summary
00:00
"Introduction to Java Programming with Fernanda Kiper"
- Fernanda Kiper introduces Java language in a video tutorial.
- The video's schedule includes understanding Java, installing it, configuring the development environment, learning basic syntax, object-oriented programming concepts, and compiling and running programs.
- Java is an object-oriented language, emphasizing data organization in objects with values and functions.
- Java is strongly typed, requiring declaration of variable types to avoid errors.
- Java is platform-independent, allowing programs to run on various machines without compatibility issues.
- The Java Virtual Machine (JVM) interprets and executes compiled Java code, ensuring platform independence.
- JVM manages memory, garbage collection, and execution of Java programs.
- To install Java, search for "Java Download Oracle," choose the version and operating system, and download the installer.
- Verify Java installation by checking the version in the terminal using "Java --version."
- Install the IntelliJ IDE for Java development, selecting the community edition for free use.
14:08
Java Data Types, Arrays, and Loops
- Declaring variables in Java involves considering the storage space required, with long types allocating 64 bits, potentially leading to wasted memory if not utilized efficiently.
- To optimize memory usage, it is advisable to use the appropriate data type, such as byte for smaller values, rather than long, which may result in unnecessary memory consumption.
- Java supports various primitive data types, including boolean for true/false values and char for single characters, with strings being non-primitive and represented as a class.
- Floating-point numbers in Java are represented by Double and float data types, with Double offering higher precision due to its larger representation range compared to float.
- Conditional statements in Java, such as if, else, and else if, follow a syntax similar to other programming languages, enabling the execution of specific actions based on defined conditions.
- Declaring arrays in Java involves specifying the data type and size, with initialization required before storing or accessing values, either directly or by using the new int Array method.
- To create dynamic arrays with flexible sizes, Java utilizes ArrayList, a class from the Java standard library that allows for the addition, removal, and retrieval of elements without specifying a fixed size.
- ArrayLists in Java require the declaration of the data type being stored, such as integer, and support methods like add, remove, and get for manipulating the array's contents.
- Understanding loops in Java, like for and while, enables repetitive actions to be performed based on specified conditions, with for loops iterating through a defined range and while loops executing until a condition is met.
- Casting in Java involves converting a value from one data type to another, with implicit casting occurring automatically and explicit casting requiring a declaration, facilitating the transformation of variables to different types as needed.
28:42
Data Type Transformation and Class Essentials in Java
- Automatic transformation from int to Double is possible due to the larger representation range of Double.
- Implicit casting from Double to int is not allowed due to the smaller representation range of int.
- Explicit casting is necessary when transforming data types, indicating awareness of potential loss of representation.
- Transformation of a character into a string requires the use of the String class and the valueOf method.
- Converting a string into a character involves specifying the position of the character in the string.
- Casting methods vary depending on the data types involved, such as chars, Strings, ints, Doubles, longs, and bytes.
- Converting an integer to a string is achieved by using the valueOf method.
- Transforming a string into an integer is possible using the parseInt method.
- Classes in Java are essential for modeling objects, defining attributes and behaviors through methods.
- Constructors in Java are methods with the same name as the class, used to initialize instances of the class. Access modifiers like Public and Private determine the visibility of classes, attributes, and methods.
44:19
Java Access Modifiers and Inheritance Basics
- The public method "getSalary" in the person class returns an integer representing the salary.
- The "getSalary" method can be accessed by the person class internally but not by external classes.
- The visibility of methods can be controlled using access modifiers like public, private, and protected.
- Changing the access modifier to protected allows classes in the same package to access the method.
- Classes from different packages cannot access protected methods unless they belong to the same package.
- Only one public class is allowed per Java file, and it must have the same name as the file.
- Inheritance allows a subclass to inherit attributes and methods from a superclass.
- Subclasses can add additional methods and attributes to those inherited from the superclass.
- Polymorphism enables objects of different classes to respond to the same message in different ways.
- Subclasses must call the constructor of the superclass when extending it, passing necessary parameters.
59:54
Polymorphism in Java: Greetings from Objects
- The new method declared will be a public method of type string, returning a string as a greeting.
- Within the "my dog" class, the method "greeting" is overridden to return "woof woof" for the dog.
- In the "person" class, the greeting method is implemented to return "Hello, my name is" followed by the name.
- Polymorphism is demonstrated as different objects from different classes respond differently to the same method call.
- The process of compiling and executing the program is detailed, including using Java compiler and IDE shortcuts for efficiency.

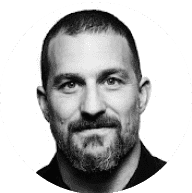
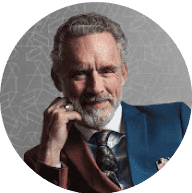
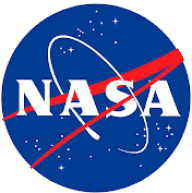
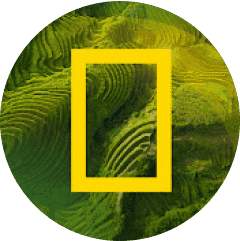