Build a TikTok Clone in React Native and AWS Backend [Tutorial for Beginners] 🔴
notJust․dev・8 minutes read
Setting up the AWS backend for a TikTok clone involves utilizing AWS Amplify for authentication, creating a GraphQL API for data storage and retrieval, and integrating AWS S3 for file storage. The process includes setting up Amplify, defining GraphQL schemas, implementing authentication, recording and uploading videos, and creating posts through GraphQL operations for a seamless user experience.
Insights
- AWS Amplify simplifies setting up authentication and GraphQL APIs for React Native applications, enhancing security and data management with pre-made screens and automatic data saving to DynamoDB.
- The GraphQL schema is crucial for defining data queries and manipulation, allowing for custom types like 'offer' and 'post' to be created, serving as a foundation for AWS Amplify to generate resources like database tables.
- Implementing features like pagination, user authentication, and video recording/uploading in React Native applications using AWS services requires careful setup, including permissions configuration, library imports, and API integration, to ensure seamless functionality and user interaction.
Get key ideas from YouTube videos. It’s free
Recent questions
How can I set up authentication for a React Native application?
To set up authentication for a React Native application, you can use AWS Amplify. Start by installing Node.js, npm, and Git, then initialize a React Native application and create an AWS account. Install the Amplify CLI globally, configure it, and initialize your project. Follow the prompts to create users, policies, and access keys. Once Amplify is initialized within your project, set up configurations through a series of questions, leading to the creation of necessary files and dependencies installation. Finally, add three lines of code in app.js to import amplify, import config, and configure amplify. This process will enable you to easily set up authentication for your React Native application using AWS Amplify.
What is the importance of the GraphQL schema in setting up a backend for an application?
The GraphQL schema is crucial in setting up a backend for an application as it defines how data will be queried and manipulated. By creating custom types like 'offer' and 'post' in the schema, you establish the structure of your data and how it can be interacted with. This schema serves as the foundation for AWS Amplify to create resources like database tables based on the defined types. With a well-defined GraphQL schema, you can ensure that your application's data is organized and accessible in a structured manner, allowing for efficient data querying and manipulation.
How can I implement pagination in a React Native application using AWS Amplify?
Implementing pagination in a React Native application with AWS Amplify involves writing a query to list posts, specifying the limit of posts to retrieve, and defining the items to receive. The next token in pagination is used for navigating to the next page of results, allowing users to access subsequent pages of data efficiently. By utilizing pagination, you can navigate through multiple pages of results seamlessly, enhancing the user experience by displaying data in a structured and organized manner.
What is the role of authentication in protecting data and connecting users in a React Native application?
Authentication plays a crucial role in protecting data and connecting users in a React Native application. By implementing authentication using services like Cognito through AWS Amplify, you can manage user access and ensure that data is secure. Amplify simplifies the authentication setup process by providing default configurations for options like username and password. By verifying user identities and linking them to their Cognito user IDs, authentication enables secure data access and interaction within the application, enhancing user privacy and data protection.
How can I upload recorded videos to AWS S3 storage in a React Native application?
To upload recorded videos to AWS S3 storage in a React Native application, you can utilize Amplify's storage service. Create a function to handle uploading a file to storage, taking a local image path as a parameter. Convert the file to a base64 version before uploading it to S3, ensuring error-free uploading by enclosing the process in a try-catch block. Use the storage.put function from AWS Amplify to upload the file to S3, specifying the file name and blob data for upload. By following these steps, you can seamlessly upload recorded videos to AWS S3 storage in your React Native application, enabling efficient data storage and retrieval.
Related videos
Summary
00:00
Setting up AWS backend for React Native
- Today's focus is on setting up the AWS backend for a React Native application, specifically a TikTok clone.
- Authentication is the first step, using AWS Amplify to easily set up pre-made screens for signing in, signing up, and more with just a few lines of code.
- Following authentication, a GraphQL API will be created using AWS Amplify to automatically save data to DynamoDB without direct interaction with the database.
- AWS S3 will be utilized to store videos and files in the cloud, simplifying the process of recording and uploading videos.
- GraphQL APIs are highlighted as an alternative to REST APIs, allowing clients to specify the data they need and easily aggregate data from various sources in a single query.
- The GraphQL schema is crucial, defining how data will be queried and manipulated, with custom types like 'offer' and 'post' being created.
- The schema is the foundation for AWS Amplify to create resources like database tables based on the defined types.
- Setting up the AWS Amplify project involves installing Node.js, npm, and Git, initializing a React Native application, and creating an AWS account.
- The Amplify CLI is globally installed, configured, and used to initialize the project, guiding users through creating users, policies, and access keys.
- Amplify is then initialized within the project, with configurations set up through a series of questions, leading to the creation of necessary files and dependencies installation.
21:25
Setting up Amplify for Front-End Applications
- To finalize the setup of libraries, add three lines of code in app.js: import amplify, import config, and configure amplify.
- In index.js, import amplify, set it up, add semicolons, and run 'yarn ios' to test the application on iOS.
- The initial compilation may take time, but subsequent runs will be faster.
- The application should run smoothly, displaying the video post page with interactive features like pausing and playing.
- The feed implementation allows smooth scrolling and snapping to different videos.
- Navigation pages like search are set up, focusing on the main video and feed features.
- Amplify is a collection of AWS services for easy integration with front-end applications.
- To add a separate service, use 'amplify add api' to set up the API locally and in the cloud.
- Choose GraphQL as the API type, set up authorization, and select a schema template for posts and users.
- Define the schema for posts, users, and songs, establish relationships, and run 'amplify push' to create resources in the cloud.
47:24
"Developed models, added data, integrated API"
- Created user, posts, and songs models for the application
- Manually created a user and multiple posts from AWS console
- Implemented queries to connect and fetch posts from the API
- Added a user with email and username, generating an ID automatically
- Ran a query to get user details by ID, specifying fields to receive
- Created a new song with a name and image URI
- Added posts by providing description, song ID, user ID, and video URI
- Ran queries to ensure posts and songs were successfully added
- Fetched a list of posts to verify data addition in the database
- Integrated API to dynamically fetch and display posts in the application
01:16:49
Efficient Pagination and Secure Authentication with Amplify
- Pagination with the API involves writing a query to list posts, specifying the limit of posts to retrieve, and defining the items to receive.
- The next token in pagination is used for navigating to the next page of results.
- Implementing pagination involves using the next token to access subsequent pages of data.
- Pagination allows for navigating through multiple pages of results efficiently.
- Authentication is crucial for protecting data and connecting it with users across devices.
- Amplify simplifies authentication setup by using Cognito for managing users.
- Amplify's default configuration for authentication includes options like username and password.
- Amplify's authentication setup involves calling 'amplify add authentication' and 'amplify push' to create necessary resources.
- Implementing authentication in the app involves importing libraries like Amplify and wrapping the app with 'withAuthenticator'.
- Amplify's authentication flow includes sign-up, confirmation code verification, and sign-in processes.
01:43:08
"User ID and Data Verification in Database"
- The user ID in the database is crucial for identifying users and linking them to their Cognito user.
- The username is not under attributes but in the data itself, making it essential for user identification.
- Checking for user info to ensure the existence of an authenticated user is a necessary step.
- Verifying the presence of a user with a specific ID in the database is vital before proceeding.
- Creating a new user involves defining their ID, username, email, and image URI.
- A random image is assigned to the user if no mechanism for uploading images is in place.
- The process involves console logging the new user data before sending it to the database.
- Checking for the existence of a user in the database and stopping execution if found is crucial.
- Installing the React Native Camera library is essential for recording and uploading videos.
- Setting up permissions for camera, microphone, and storage on both iOS and Android platforms is necessary for the library to function correctly.
02:16:58
Implementing Touchable Opacity for Recording Button
- To implement touchable opacity, import it and define it as a function called onRecord.
- Set the button's height to 50 pixels, width to 50 pixels, and background color to white, to be changed based on recording status.
- Adjust the button's styling with margin vertical of 10 pixels on top and bottom.
- To make the button round, set the border radius to half of the width and height, like 25 pixels.
- Create two button styles, 'buttonRecord' and 'buttonStop', based on the 'isRecording' state variable.
- Use a reference to the camera to start and stop recording, with 'camera.current' for reference.
- Implement callback functions 'onRecordingStart' and 'onRecordingEnd' from the react native camera library for recording status.
- Create a new screen 'Create Post' for users to add a caption to the recorded video and upload it to the database.
- Define a text input for the description and a button for publishing the video.
- Style the text input with a height of 150 pixels, white background, and placeholder text.
- Style the button with a pink background, centered text, and bold white font.
- Align the button at the bottom of the screen with margins and height adjustments.
- Add functionality to upload the recorded video to AWS S3 storage using Amplify's storage service.
- Create a function 'uploadToStorage' to handle uploading a file to the storage, taking a local image path as a parameter.
02:54:43
"Efficient S3 File Upload Process with AWS"
- To ensure error-free uploading, enclose the process in a try-catch block to handle potential issues that could disrupt the replication process.
- Before sending a file to S3, convert it to a base64 version by fetching the image path and extracting the blob version using the fetch function.
- Utilize the blob file obtained to upload to S3 by employing the storage.put function, specifying the file name and the blob data for upload.
- Import the storage module from AWS Amplify to facilitate the file upload process to S3.
- Retrieve the image path from route parameters, specifically the video URI, to initiate the upload process.
- Implement the useEffect hook to trigger the upload process with the file URL upon mounting the component.
- Generate a unique file name using the uuid library to ensure each uploaded file has a distinct identifier.
- Capture the key from the S3 response to store it for future reference, such as linking it to the database entry for the post.
- Create a new post by utilizing the API and GraphQL operations, including the necessary input data like video URI, description, user ID, and song ID.
- Implement navigation to redirect to the home screen upon successful upload and post creation, ensuring a seamless user experience.
03:28:16
"Feedback on TikTok features and Amplify advice"
- Viewers are thanked for watching the long live stream and encouraged to provide feedback on whether to continue with TikTok features like comments, video filters, and liking pagination, or start a new project. Suggestions for cloning projects are welcomed.
- Advice is given to a viewer stuck on the Amplify part of a Twitter clone project, recommending checking out documentation, free tutorials, and planning specific short tutorials on Amplify features. Encouragement is provided to viewers to continue building on the project by adding features like song selection, likes, comments, shares, and profile picture editing based on the existing functionalities demonstrated in the videos.

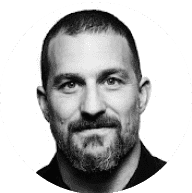
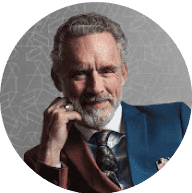
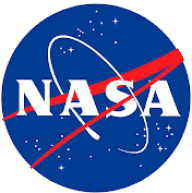
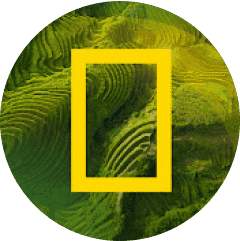