5.1 Graph Traversals - BFS & DFS -Breadth First Search and Depth First Search
Abdul Bari・2 minutes read
Breadth-first search (BFS) and depth-first search (DFS) are two graph traversal methods, with BFS exploring all adjacent vertices systematically using a queue, while DFS uses a stack to explore as deeply as possible along one branch before backtracking. Both methods have a time complexity of O(n) and offer flexibility in traversal paths, with BFS resembling level-order traversal and DFS resembling pre-order traversal in binary trees.
Insights
- Breadth-first search (BFS) systematically explores all adjacent vertices of a starting vertex before moving deeper, utilizing a queue to manage the order of exploration, which ensures that all vertices are visited in a level-order fashion similar to that of a binary tree.
- Depth-first search (DFS) dives deep into one branch of the graph using a stack, visiting vertices until it can no longer proceed, at which point it backtracks to explore other paths, resembling a pre-order traversal of a binary tree and providing a different approach to graph exploration.
Get key ideas from YouTube videos. It’s free
Recent questions
What is breadth-first search?
Breadth-first search (BFS) is a graph traversal algorithm that explores all the neighboring vertices of a given vertex before moving on to the next level of vertices. It starts from a selected vertex and systematically visits all adjacent vertices, ensuring that each vertex is explored in layers. This method is particularly useful for finding the shortest path in unweighted graphs, as it guarantees that the first time a vertex is reached, it is done so via the shortest possible route. BFS is typically implemented using a queue data structure, where the initial vertex is added to the queue, and the algorithm continues to dequeue vertices, exploring their neighbors and adding them to the queue until all vertices have been visited.
How does depth-first search work?
Depth-first search (DFS) is a graph traversal technique that explores as far down a branch as possible before backtracking. Starting from a selected vertex, DFS visits an adjacent vertex and continues to explore deeper into the graph until it reaches a vertex with no unvisited adjacent vertices. At this point, the algorithm backtracks to explore other branches. This method can be implemented using a stack data structure, where the current vertex is pushed onto the stack, and exploration continues until all paths have been explored. DFS is particularly useful for tasks such as topological sorting and finding connected components in a graph.
What is the difference between BFS and DFS?
The primary difference between breadth-first search (BFS) and depth-first search (DFS) lies in their exploration strategies. BFS explores all adjacent vertices at the present depth level before moving on to vertices at the next depth level, effectively traversing the graph in layers. In contrast, DFS dives deep into one branch of the graph until it can go no further, at which point it backtracks to explore other branches. This results in different traversal orders and can affect the performance and outcomes of algorithms that rely on these methods. BFS is often used for finding the shortest path in unweighted graphs, while DFS is useful for exploring all possible paths and structures within a graph.
What data structures are used in BFS and DFS?
Breadth-first search (BFS) utilizes a queue data structure to manage the vertices that need to be explored. When a vertex is visited, its adjacent vertices are added to the queue, ensuring that they are explored in the order they were discovered. This FIFO (first-in, first-out) approach allows BFS to systematically explore all vertices at the current level before moving deeper. On the other hand, depth-first search (DFS) employs a stack data structure, which can be implemented using recursion. In DFS, when a vertex is visited, the algorithm suspends its exploration to delve deeper into a newly discovered vertex, allowing it to backtrack when necessary. This LIFO (last-in, first-out) approach enables DFS to explore paths thoroughly before returning to previous vertices.
What is the time complexity of BFS and DFS?
The time complexity of both breadth-first search (BFS) and depth-first search (DFS) is O(n), where n represents the number of nodes in the graph. This means that the time taken to complete the traversal is proportional to the number of vertices present. In both algorithms, each vertex is visited once, and the edges are examined, leading to a linear relationship between the number of nodes and the time required for traversal. This efficiency makes both BFS and DFS suitable for various applications in graph theory, such as pathfinding, connectivity checks, and exploring graph structures.
Related videos
Summary
00:00
Graph Traversal Methods BFS and DFS Explained
- Breadth-first search (BFS) and depth-first search (DFS) are graph traversal methods, with BFS exploring all adjacent vertices before moving deeper, while DFS explores as far as possible along one branch before backtracking.
- In BFS, starting from vertex 1, adjacent vertices 2, 4, and 5 are visited in any order; for example, visiting 2 first, then 4, and finally 5.
- Exploration in BFS involves visiting all adjacent vertices of a vertex before moving to the next unvisited vertex, ensuring all vertices are explored systematically.
- In DFS, starting from vertex 1, the exploration goes deeper by visiting vertex 2, then 3, and continuing until no adjacent vertices remain, before backtracking to explore other branches.
- BFS can be implemented using a queue data structure, where the initial step involves adding the starting vertex to the queue and exploring its adjacent vertices.
- The repeating step in BFS involves dequeuing a vertex, exploring its adjacent vertices, and adding them to the queue until all vertices are visited.
- DFS uses a stack data structure, where the initial step involves visiting a vertex and suspending its exploration to explore a newly visited vertex, continuing until all paths are explored.
- In DFS, once a vertex is visited, exploration of its adjacent vertices is suspended, allowing the algorithm to backtrack and explore other vertices when necessary.
- Both BFS and DFS allow starting from any vertex and visiting adjacent vertices in any order, providing flexibility in traversal paths.
- The results of BFS resemble level-order traversal of a binary tree, while DFS resembles pre-order traversal, highlighting the structural differences in their exploration methods.
18:23
Time Complexity of DFS and BFS Explained
- The time complexity of depth-first search (DFS) and breadth-first search (BFS) is O(n), where n represents the number of nodes in the graph.

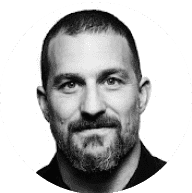
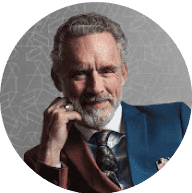
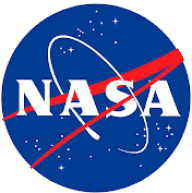
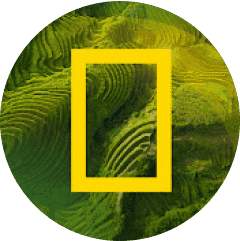