4 JavaScript Projects under 4 Hours | JavaScript Projects For Beginners | JavaScript | Simplilearn
Simplilearn・2 minutes read
Mastering a language involves programming from simple to advanced projects covering a calculator app, weather app, tic-tac-toe, and snake game. Detailed coding instructions for each project are provided to create functional applications, with a focus on JavaScript development and CSS styling.
Insights
- Mastering a language involves starting with simple projects like a calculator app and progressing to more advanced projects like a weather app, tic-tac-toe game, and snake game.
- Detailed instructions on creating each project, from setting up the project structure to implementing JavaScript functions for user interaction and game logic.
- The importance of CSS styling in enhancing the visual appeal of applications, with specific properties and classes defined for layout, buttons, and game interfaces.
Get key ideas from YouTube videos. It’s free
Recent questions
How can I create a calculator app?
To create a calculator app, start by making a new folder named "calculator app" with index.html, app.css, and app.js files. Structure the index.html file with an h1 tag for the calculator title and a table for organizing elements. Create buttons for numbers, operators, and functions like clear and solve within the table. Apply CSS styling to elements like the screen, buttons, and container for a visually appealing layout. Implement JavaScript functions like display and solve to handle user input and perform calculations. Execute the code to ensure the calculator application functions correctly.
What is involved in making a weather app?
Making a weather app involves creating HTML, CSS, and JavaScript files named "index.html," "app.css," and "app.js" respectively. You will make asynchronous calls to a REST API for weather information. Define CSS properties for elements like temperature description, location, and div positioning. Use JavaScript to select elements, get input field data, and interact with the OpenWeatherMap API. The Fetch API retrieves weather data, which you display on the application. Ensure to handle cases where weather and location data are not found.
How do I develop a Tic-Tac-Toe game?
To develop a Tic-Tac-Toe game, create an index.html file with div elements for the game, player input fields, and a submit button. Design div elements for the game board and a replay button. Implement JavaScript logic for the game, including initializing variables, adding players, and determining the current player. Functions for resizing squares on browser resize events are essential. Create a separate app.js file for the JavaScript logic of the game, including functions for gameplay functionality and resetting the board.
What steps are involved in creating a snake game?
Creating a snake game involves setting up variables for game elements like the canvas, snake, and game speed in the initialization function of JavaScript. Define the game area, media context, width, and height. Establish game start logic, player score, snake direction, and speed size based on user input. Implement functions for game logic, interval clearing, food creation, and changing directions. Test the game in the browser, ensuring CSS styling enhances the game's appearance.
How can I improve the appearance of a game interface?
To enhance the appearance of a game interface, add CSS styling to the HTML file with properties like font size, text alignment, padding, background color, and border radius. Ensure the CSS content improves the visual appeal of the game. Test the game in the browser, making necessary adjustments to naming issues and styling to create an engaging user experience. Include interactive elements like a "start" button that enables gameplay features like direction changes, scoring, automatic food creation, and snake growth.
Related videos
Error Makes Clever
Python Tutorial - Python Full Course for Beginners in Tamil
College Wallah
C Programming in One Shot | Part 1 | Variables, Operators and Input/ Output | C Complete Course
Web Dev Simplified
Build A Calculator With JavaScript Tutorial
Thomas Frank
I learned to code from scratch in 1 year. Here's how.
Fireship
God-Tier Developer Roadmap
Summary
00:00
Creating Calculator and Snake Game Applications
- Mastering a language involves programming in it, starting with simple projects like a calculator app.
- Advanced projects like a weather app, tic-tac-toe game, and snake game will be covered.
- The session will conclude with creating a snake game application.
- The process begins by creating a new folder named "calculator app" with index.html, app.css, and app.js files.
- The index.html file is structured with an h1 tag for the calculator title and a table for organizing elements.
- Buttons for numbers, operators, and functions like clear and solve are created within the table.
- CSS styling is applied to elements like the screen, buttons, and container for a visually appealing layout.
- Specific CSS properties like background color, padding, border, and cursor are defined for different button types.
- The container's width is set to 40%, with styling for alignment, border radius, padding, and box shadow.
- JavaScript functions like display and solve are implemented to handle user input and perform calculations in the calculator app.
29:19
Creating Interactive Calculator and Weather App
- To retrieve a value, use "let x" to get the element by ID "result" and assign it to "x".
- Calculate "y" using a function named "libra" with the parameters "axis".
- Set the value of the element with ID "result" to "y".
- Implement a function named "clear screen" to set the value of the element with ID "result" to empty.
- Three functions, including "libra" and "clear screen," are part of a JavaScript file named "app.js".
- Execute the code to perform calculations like "x + 6 = 8" and "6 / 2 = 3".
- The calculator application is now functional and ready for use.
- A new project, "WeatherPro," is introduced, focusing on real-time weather data.
- The project involves making asynchronous calls to a REST API for weather information.
- Development includes creating HTML, CSS, and JavaScript files named "index.html," "app.css," and "app.js" respectively.
57:47
CSS and JavaScript for Weather Display
- CSS properties for temperature description: padding of 8 pixels, margin 0, color, text-align center, font size 1.2.
- CSS properties for location: margin 0, padding 0, color, text-align center, font size 0.8.
- CSS properties for a div: position relative, absolute top at 600 pixels, right at 120 pixels.
- Introduction of CSS classes in HTML: autocomplete off, temperature value, location icon, notification, weather condition, weather icon, location, temperature value, location.
- Script source added to HTML: app.js.
- Notification displayed when weather and location not found.
- JavaScript code to select elements: icon element, location icon, temp element, desc element, location element, notification element.
- JavaScript code to get input field and coordinates: input field with id search, city, latitude, longitude.
- JavaScript code to interact with OpenWeatherMap API: API key obtained, geolocation accessed, position set, weather data fetched.
01:26:54
Creating Weather App & JavaScript Game Tutorial
- The text discusses coding instructions for a weather application, focusing on variables like latitude, longitude, and app ID.
- It mentions using the Fetch API to retrieve weather data and display it on the application.
- Specific coding steps are detailed, such as setting the icon element, temperature element, description element, and location element.
- The text then transitions to discussing a free learning opportunity on Scale Up by Simply Learn.
- It introduces the development of a JavaScript game, Tic-Tac-Toe, with instructions to create an index.html file and div elements for the game.
- The text outlines the creation of player input fields and a submit button for the game.
- It further details the creation of div elements for the game board and a replay button.
- The text then delves into the JavaScript logic for the game, including initializing variables, adding players, and determining the current player.
- It includes functions for resizing squares on browser resize events.
- The text concludes by mentioning the need to create a separate app.js file for the JavaScript logic of the game.
01:56:10
Dynamic Cell Height and Game Functionality
- Create a function called `onResize` that sets the height of all cells dynamically.
- Use `document.querySelectorAll` to select all cells on the board.
- Calculate the height of cells using `offsetWidth`.
- Set the height of each cell using a template literal with the dynamic height value.
- Launch the `buildBoard` function to create the board.
- Define the `buildBoard` function to reset the container and remove the `reset-hidden` class.
- Call the `onResize` and `addCellClickListener` functions.
- Implement the `addCellClickListener` function for player 2 to make a move.
- Check if the cell is empty and assign the current player's token.
- Check for a winner using the `isWinner` function and update the turn.
- Check for a tie and end the game if necessary.
- Create a function to check winning sequences and highlight the winning cells.
- Update the player's turn and display a message for the winner.
- Implement a function to reset the board and update the player's turn.
- Add and remove cell click listeners for gameplay functionality.
- Set basic styling for the body, including background image properties.
02:29:26
Styling Game Interface with CSS and JavaScript
- The CSS properties for a game interface are detailed, including pixel measurements and color choices.
- Specific classes like "inter-player" and "submit-btn" are defined with their respective styles.
- Instructions for input fields and their focus states are provided, specifying border styles and padding.
- Styling for the submit button is outlined, including border, padding, font size, width, and background color.
- Additional styles for the submit button's active and hover states are detailed, with changes in border color and background color.
- Classes for player and board elements are mentioned, with color and background settings.
- The process of including a custom font class, "permanent marker," using an external API is explained.
- The HTML structure for a game interface is described, including input fields, buttons, and canvas elements.
- The initialization function in JavaScript is outlined, setting up variables for game elements like the canvas, snake, and game speed.
- The process of setting up the game interface and initializing game elements is detailed, preparing for further development of a snake game project.
03:02:50
"Game setup and logic for snake game"
- Values include game start and game speed.
- Game speed is set using document.getElementById.
- Game area value is defined.
- Game media context is set to 2D.
- Game area width and height are initialized.
- Logic for game start on click is defined.
- Initial player score is set to zero.
- Snake direction is set to right.
- Speed size is determined based on user input.
- Functions for game logic, interval clearing, and food creation are outlined.
03:31:31
"Snake Game Tutorial with CSS Styling"
- A function named "change direction" is defined, taking a parameter "e" and utilizing an option called "workkeys" set to "e".
- If "keys" equal 40 and "snake direction" equals "up", the direction is changed to "tau".
- The function includes four "elseif" statements, with values of 30, 40, 39, 38, 37, 39, 38, 27, and directions of up, left, down, and right.
- The script includes a call to "window.onkeydown" for "change directions" and "window.onload" for "initialize".
- CSS styling is added in "app.css" to enhance the appearance of the game, including font, margin, background, height, and other visual elements.
- The game is tested in the browser, showing the need for CSS content to improve the appearance.
- CSS styling is added to the HTML file, including font size, text alignment, padding, background color, and border radius.
- The game is relaunched after fixing some naming issues, allowing for gameplay with direction changes and scoring.
- The game includes a "start" button that disables until the game is finished, with automatic food creation and snake growth.
- The tutorial concludes, encouraging feedback and further learning opportunities from Cynthia.

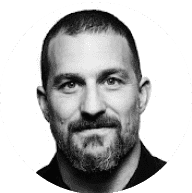
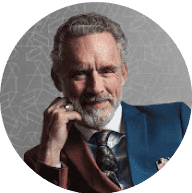
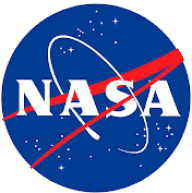
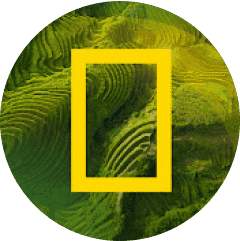